#include<stdio.h> #include<stdlib.h> #include <unistd.h> #include<sys/wait.h> #include<sys/types.h> int main () { pid_t pida,pidb,pidc,pidd; while((pidb=fork())==-1); if(pidb>0) { wait(0); printf("Apid=%d,ppid=%d\n",getpid(),getppid()); } else //p { while((pidc=fork())==-1); if(pidc>0) { wait(0); printf("Bpid=%d,pidd=%d\n",getpid(),getppid()); } else //p { while((pidd=fork())==-1); if(pidd >0) //p2 { wait(0); printf("Cpid=%d,pidd=%d\n",getpid(),getppid()); } else //p { printf("Dpid=%d,pidd=%d\n",getpid(),getppid()); } } } }
时间: 2024-02-07 15:02:52 浏览: 30
这是一个使用了fork()函数的进程创建程序,它会创建4个进程(A、B、C、D)。下面是程序的大致流程:
1. 主进程先创建一个子进程B(pidb=fork()),如果失败则一直循环直到成功为止。
2. 如果是父进程,等待子进程B结束(wait(0)),然后输出A进程的pid和ppid。
3. 如果是子进程B,创建一个子进程C,如果失败则一直循环直到成功为止。
4. 如果是父进程B,等待子进程C结束(wait(0)),然后输出B进程的pid和ppid。
5. 如果是子进程C,创建一个子进程D,如果失败则一直循环直到成功为止。
6. 如果是父进程C,等待子进程D结束(wait(0)),然后输出C进程的pid和ppid。
7. 如果是子进程D,直接输出D进程的pid和ppid。
8. 程序结束。
总体来说,这个程序的输出结果是不确定的,因为进程的调度是由操作系统决定的,不同的操作系统或者不同的时刻都可能会产生不同的输出结果。
相关问题
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/wait.h> int main( ) { int fd[2]; char buf[30], s[30]; pipe(fd); //创建管道 while((fork()) == -1); //创建子程序失败时,循环 if(fork() == 0) //子进程返回 { sprintf(buf, "This is an example\n"); //将 buf 中字符写入管道 write(fd[1], buf, sizeof(buf)); //将 buf 中字符写入管道 } else //父进程返回 { wait(0); //等待子程序结束 read(fd[0], s, sizeof(s)); //父进程读管道中字符 printf("%s", s); //输出读取到的字符 } return 0; }改良以上程序
以上程序是一个简单的管道通信例子,可以通过管道将一个字符串从子进程传递给父进程并输出。以下是对程序的改良:
1. 错误处理:以上程序没有进行错误处理,可以在创建管道和创建子进程时添加错误处理语句。
2. 参数传递:以上程序只传递了一个字符串,可以通过参数传递实现更加灵活的通信。
3. 程序退出:以上程序没有使用 exit() 函数退出程序,可以添加退出语句以保证程序正常结束。
改良后的程序如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main(int argc, char *argv[]) {
int fd[2];
char buf[30], s[30];
if (pipe(fd) == -1) { //创建管道失败
perror("pipe");
exit(EXIT_FAILURE);
}
pid_t pid = fork();
if (pid == -1) { //创建子进程失败
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid == 0) { //子进程返回
if (close(fd[0]) == -1) { //关闭管道读端
perror("close");
exit(EXIT_FAILURE);
}
sprintf(buf, "%s\n", argv[1]); //将参数写入管道
if (write(fd[1], buf, sizeof(buf)) == -1) { //将 buf 中字符写入管道
perror("write");
exit(EXIT_FAILURE);
}
if (close(fd[1]) == -1) { //关闭管道写端
perror("close");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
else { //父进程返回
if (close(fd[1]) == -1) { //关闭管道写端
perror("close");
exit(EXIT_FAILURE);
}
if (read(fd[0], s, sizeof(s)) == -1) { //父进程读管道中字符
perror("read");
exit(EXIT_FAILURE);
}
printf("%s", s); //输出读取到的字符
if (close(fd[0]) == -1) { //关闭管道读端
perror("close");
exit(EXIT_FAILURE);
}
wait(NULL); //等待子程序结束
exit(EXIT_SUCCESS);
}
return 0;
}
```
改良后的程序增加了错误处理和参数传递功能,程序退出时使用了 exit() 函数。
/* Socket head file */ #include<sys/types.h> #include<sys/socket.h> #include<netinet/in.h>/*sockaddr_in{}*/ #include<arpa/inet.h> /* Unix standard head file */ #include<stdio.h> #include<stdlib.h> #include<string.h> #include<unistd.h> #include <errno.h>/* strerror(),perror(),errno head file*/ #include <sys/time.h> #include <sys/wait.h> #define MAXBUFF 1024 #define PORT 9999怎么改成windows系统
在Windows系统中,网络编程需要使用Winsock API,因此需要引入`<winsock2.h>`头文件。此外,需要在程序中调用`WSAStartup()`函数和`WSACleanup()`函数来初始化和释放Winsock库。
下面是在Windows系统下进行网络编程的头文件和常量定义示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <winsock2.h>
#include <ws2tcpip.h>
#pragma comment(lib, "Ws2_32.lib")
#define MAXBUFF 1024
#define PORT 9999
```
其中,`<ws2tcpip.h>`头文件包含了一些网络编程相关的函数和结构体定义。`#pragma comment(lib, "Ws2_32.lib")`语句用于链接Winsock库。
需要注意的是,在Windows系统中,端口号的类型为`unsigned short`,而不是`int`。因此,定义端口号常量时应该使用`USHORT`类型:
```c
#define PORT (USHORT)9999
```
相关推荐
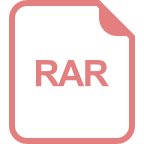
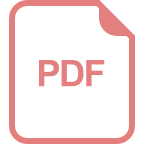
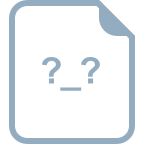













