使用python写一个mcts算法
时间: 2023-06-13 10:08:42 浏览: 51
好的,下面是一个简单的Python实现MCTS算法的示例:
```python
import math
import random
# 定义一个节点类
class Node:
def __init__(self, state, parent=None):
self.state = state
self.parent = parent
self.children = []
self.visits = 0
self.score = 0.0
def add_child(self, child_state):
child = Node(child_state, self)
self.children.append(child)
return child
def update(self, score):
self.visits += 1
self.score += score
def fully_expanded(self):
return len(self.children) == len(self.state.get_legal_moves())
def __repr__(self):
return f"Node(visits={self.visits}, score={self.score})"
# 定义一个MCTS类
class MCTS:
def __init__(self, time_limit=None, exploration_constant=1 / math.sqrt(2)):
self.time_limit = time_limit
self.exploration_constant = exploration_constant
def search(self, initial_state):
self.root = Node(initial_state)
start_time = datetime.now()
while True:
node = self.select(self.root)
if node is None:
break
if node.state.is_terminal():
score = node.state.get_score()
else:
child = self.expand(node)
if child is None:
score = 0
else:
score = self.simulate(child.state)
child.update(score)
self.backpropagate(node, score)
elapsed_time = datetime.now() - start_time
if self.time_limit is not None and elapsed_time > self.time_limit:
break
best_child = self.best_child(self.root)
return best_child.state.get_last_move()
def select(self, node):
while not node.state.is_terminal():
if not node.fully_expanded():
return node
node = self.best_child(node)
return None
def best_child(self, node):
best_score = float("-inf")
best_children = []
for child in node.children:
score = child.score / child.visits + self.exploration_constant * math.sqrt(
2 * math.log(node.visits) / child.visits
)
if score == best_score:
best_children.append(child)
elif score > best_score:
best_children = [child]
best_score = score
return random.choice(best_children)
def expand(self, node):
untried_moves = [move for move in node.state.get_legal_moves() if move not in node.children]
if not untried_moves:
return None
move = random.choice(untried_moves)
child_state = node.state.apply_move(move)
return node.add_child(child_state)
def simulate(self, state):
while not state.is_terminal():
move = random.choice(state.get_legal_moves())
state = state.apply_move(move)
return state.get_score()
def backpropagate(self, node, score):
while node is not None:
node.update(score)
node = node.parent
```
这是一个基本的MCTS算法实现,但是需要注意的是,这个示例只是为了展示MCTS算法的基本操作,实际应用中需要根据具体情况进行修改和优化。
相关推荐
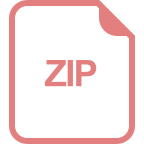














