写一个c++贪吃蛇游戏代码
时间: 2023-10-29 19:10:06 浏览: 81
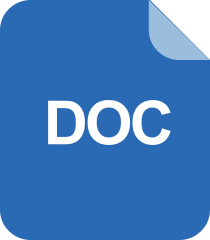
贪吃蛇游戏代码(C++)
下面是一个简单的 C 语言贪吃蛇游戏代码,供参考:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <time.h>
#include <windows.h>
#define ROW 20
#define COL 30
int snake[100][2]; // 蛇
int head, tail; // 蛇头和蛇尾
int food[2]; // 食物
int score; // 得分
void init(); // 初始化游戏
void draw(); // 绘制游戏界面
void createFood(); // 随机生成食物
void update(); // 更新蛇的位置和状态
int main() {
init();
while (1) {
draw();
update();
Sleep(100); // 休眠100毫秒,控制蛇移动速度
}
return 0;
}
void init() {
srand(time(NULL));
// 初始化蛇
snake[0][0] = ROW / 2;
snake[0][1] = COL / 2;
head = 0;
tail = 0;
// 初始化食物
createFood();
// 初始化得分
score = 0;
}
void draw() {
system("cls"); // 清屏
// 绘制上边界
for (int i = 0; i < COL + 2; i++) {
printf("#");
}
printf("\n");
// 绘制中间部分
for (int i = 0; i < ROW; i++) {
printf("#"); // 左边界
for (int j = 0; j < COL; j++) {
if (i == snake[head][0] && j == snake[head][1]) {
printf("@"); // 绘制蛇头
} else if (i == food[0] && j == food[1]) {
printf("*"); // 绘制食物
} else {
int flag = 0;
for (int k = tail; k <= head; k++) {
if (i == snake[k][0] && j == snake[k][1]) {
printf("#"); // 绘制蛇身
flag = 1;
break;
}
}
if (!flag) {
printf(" "); // 空格
}
}
}
printf("#\n"); // 右边界
}
// 绘制下边界
for (int i = 0; i < COL + 2; i++) {
printf("#");
}
printf("\n");
// 绘制得分
printf("Score: %d\n", score);
}
void createFood() {
// 随机生成食物
int row = rand() % ROW;
int col = rand() % COL;
int flag = 0;
for (int i = 0; i <= head; i++) {
if (row == snake[i][0] && col == snake[i][1]) {
flag = 1;
break;
}
}
if (flag) {
createFood();
} else {
food[0] = row;
food[1] = col;
}
}
void update() {
// 检测键盘输入
if (_kbhit()) {
char ch = _getch();
switch (ch) {
case 'w': // 向上
if (head == tail || (snake[head][0] - 1) != snake[head - 1][0]) {
head++;
snake[head][0] = snake[head - 1][0] - 1;
snake[head][1] = snake[head - 1][1];
}
break;
case 's': // 向下
if (head == tail || (snake[head][0] + 1) != snake[head - 1][0]) {
head++;
snake[head][0] = snake[head - 1][0] + 1;
snake[head][1] = snake[head - 1][1];
}
break;
case 'a': // 向左
if (head == tail || (snake[head][1] - 1) != snake[head - 1][1]) {
head++;
snake[head][0] = snake[head - 1][0];
snake[head][1] = snake[head - 1][1] - 1;
}
break;
case 'd': // 向右
if (head == tail || (snake[head][1] + 1) != snake[head - 1][1]) {
head++;
snake[head][0] = snake[head - 1][0];
snake[head][1] = snake[head - 1][1] + 1;
}
break;
}
} else { // 没有键盘输入
head++;
snake[head][0] = snake[head - 1][0];
snake[head][1] = snake[head - 1][1] + 1;
}
// 检测是否撞墙
if (snake[head][0] < 0 || snake[head][0] >= ROW || snake[head][1] < 0 || snake[head][1] >= COL) {
printf("You Lose!\n");
exit(0);
}
// 检测是否吃到食物
if (snake[head][0] == food[0] && snake[head][1] == food[1]) {
tail--;
score++;
createFood();
} else {
tail++;
}
// 检测是否撞到自己的身体
for (int i = tail; i < head; i++) {
if (snake[head][0] == snake[i][0] && snake[head][1] == snake[i][1]) {
printf("You Lose!\n");
exit(0);
}
}
}
```
这只是一个简单的贪吃蛇游戏,实现了基本的游戏功能,您可以根据需求进行更改和优化。
阅读全文
相关推荐
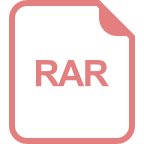


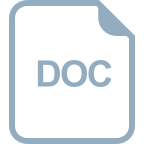
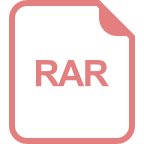
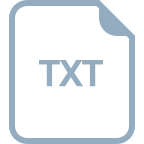
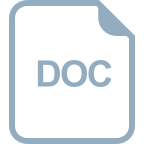
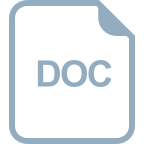



