使用GPU对这段python代码进行加速
时间: 2024-02-03 18:03:49 浏览: 27
要使用GPU对Python代码进行加速,可以使用CUDA编程语言,或使用现有的GPU加速库,如Numba或PyCUDA。
以下是使用Numba对Python代码进行GPU加速的示例:
```python
from numba import cuda
import numpy as np
# 定义GPU核函数
@cuda.jit
def split_intersect(mask1, mask2, intersect, line_points, line_y, line_x):
i, j = cuda.grid(2)
if i < mask1.shape[0] and j < mask1.shape[1]:
if i < np.min(line_points, axis=0)[1] or i > np.max(line_points, axis=0)[1]:
return
else:
y_index = np.where(line_y == i)[0][0]
if j < line_x[y_index]:
mask1[i][j] = True
mask2[i][j] = False
else:
mask1[i][j] = False
mask2[i][j] = True
# 定义主函数
def main():
# 初始化输入数据
intersect = np.array([[0, 1, 1], [1, 0, 1], [1, 1, 0]], dtype=np.bool)
mask1 = intersect.copy()
mask2 = intersect.copy()
line_points = np.array([[0, 1], [1, 0]], dtype=np.int32)
line_y = np.array([0, 1], dtype=np.int32)
line_x = np.array([1, 0], dtype=np.int32)
# 将数据传输到GPU内存中
d_mask1 = cuda.to_device(mask1)
d_mask2 = cuda.to_device(mask2)
d_intersect = cuda.to_device(intersect)
d_line_points = cuda.to_device(line_points)
d_line_y = cuda.to_device(line_y)
d_line_x = cuda.to_device(line_x)
# 定义GPU线程块和线程数
threadsperblock = (16, 16)
blockspergrid_x = np.ceil(mask1.shape[0] / threadsperblock[0]).astype(np.int32)
blockspergrid_y = np.ceil(mask1.shape[1] / threadsperblock[1]).astype(np.int32)
blockspergrid = (blockspergrid_x, blockspergrid_y)
# 调用GPU核函数进行计算
split_intersect[blockspergrid, threadsperblock](d_mask1, d_mask2, d_intersect, d_line_points, d_line_y, d_line_x)
# 将计算结果从GPU内存中传输回CPU内存中
mask1 = d_mask1.copy_to_host()
mask2 = d_mask2.copy_to_host()
# 输出结果
print("mask1:")
print(mask1)
print("mask2:")
print(mask2))
if __name__ == '__main__':
main()
```
这里使用了 `numba.cuda.jit` 装饰器来定义GPU核函数 `split_intersect`。在主函数中,先将输入数据传输到GPU内存中,然后定义GPU线程块和线程数,并调用GPU核函数进行计算。最后,将计算结果从GPU内存中传输回CPU内存中,输出结果。
需要注意的是,GPU加速并不是万能的,只有部分算法能够受益于GPU加速。在实际应用中,需要根据算法的特点和数据的规模来选择是否使用GPU加速。
相关推荐
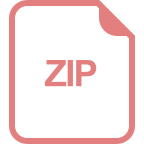
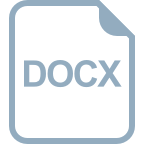
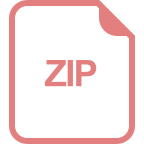
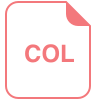













