servlet实现将商品加入到mysql数据库表中,并且判断用户是否是mysql数据库user表中已经登陆的用户,如果登陆执行加入购物车,如果没有返回用户登陆页面,并且判断加入的商品是否购物车已经有,如果有数量加一,没有就加入
时间: 2024-01-29 12:04:49 浏览: 20
要实现将商品加入到MySQL数据库表中,并判断用户是否已经登录,以及商品是否已经存在于购物车中,您可以按照以下步骤进行操作:
1.编写一个servlet来处理用户的登录请求。您可以从请求对象中获取用户输入的用户名和密码,然后将其与MySQL数据库中的用户表进行比较。如果用户存在,将其添加到会话中,以便在后续请求中使用。
2.编写一个servlet来处理添加商品到购物车的请求。从请求对象中获取商品信息,并检查会话中是否有用户已经登录。如果用户已经登录,则检查购物车中是否已经存在该商品。如果存在,则将其数量加一,否则将其添加到购物车中。
下面是一个示例代码,您可以根据自己的需求进行修改:
```java
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
import java.sql.*;
public class LoginServlet extends HttpServlet {
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取表单数据
String username = request.getParameter("username");
String password = request.getParameter("password");
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
// 注册JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 打开连接
conn = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password");
// 执行查询语句
String sql = "SELECT * FROM user WHERE username = ? AND password = ?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, password);
rs = stmt.executeQuery();
// 判断用户是否存在
if (rs.next()) {
// 将用户添加到会话中
HttpSession session = request.getSession();
session.setAttribute("username", username);
// 返回成功信息
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Login Successful</h2>");
out.println("</body></html>");
} else {
// 返回错误信息
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Invalid Username or Password</h2>");
out.println("</body></html>");
}
} catch(Exception e) {
// 返回错误信息
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Error Logging in: " + e.getMessage() + "</h2>");
out.println("</body></html>");
} finally {
// 关闭连接、声明和结果集
try {
if (rs != null) rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (stmt != null) stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
public class AddToCartServlet extends HttpServlet {
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取表单数据
String productName = request.getParameter("productName");
String productDescription = request.getParameter("productDescription");
double productPrice = Double.parseDouble(request.getParameter("productPrice"));
HttpSession session = request.getSession();
String username = (String) session.getAttribute("username");
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
// 注册JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 打开连接
conn = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password");
// 检查购物车中是否已经存在该商品
String sql = "SELECT * FROM cart WHERE username = ? AND name = ?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, productName);
rs = stmt.executeQuery();
if (rs.next()) {
// 商品已经存在,将其数量加一
int quantity = rs.getInt("quantity") + 1;
sql = "UPDATE cart SET quantity = ? WHERE username = ? AND name = ?";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, quantity);
stmt.setString(2, username);
stmt.setString(3, productName);
stmt.executeUpdate();
} else {
// 商品不存在,将其添加到购物车中
sql = "INSERT INTO cart (username, name, description, price, quantity) VALUES (?, ?, ?, ?, ?)";
stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, productName);
stmt.setString(3, productDescription);
stmt.setDouble(4, productPrice);
stmt.setInt(5, 1);
stmt.executeUpdate();
}
// 返回成功信息
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Product Added to Cart Successfully</h2>");
out.println("</body></html>");
} catch(Exception e) {
// 返回错误信息
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h2>Error Adding Product to Cart: " + e.getMessage() + "</h2>");
out.println("</body></html>");
} finally {
// 关闭连接、声明和结果集
try {
if (rs != null) rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (stmt != null) stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
try {
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的代码中,我们首先编写了一个用于处理用户登录请求的servlet。如果用户存在,则将其添加到会话中以便在后续请求中使用。接下来,我们编写了一个用于处理添加商品到购物车的请求的servlet。我们从请求对象中获取商品信息,并检查会话中是否有用户已经登录。如果用户已经登录,则检查购物车中是否已经存在该商品。如果存在,则将其数量加一,否则将其添加到购物车中。
请注意,您需要将MySQL连接信息中的“mydatabase”替换为您要连接的实际数据库名称,以及“username”和“password”替换为您的MySQL用户名和密码。
相关推荐
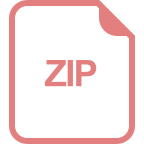
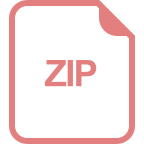
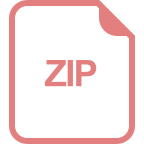














