matlab通过fopen实现写入PPM图像P6的功能
时间: 2023-10-08 16:12:51 浏览: 40
以下是通过 MATLAB 的 fopen 函数实现写入 PPM 图像 P6 的示例代码:
```matlab
% 定义图像宽度、高度和颜色深度
width = 640;
height = 480;
maxColor = 255;
% 创建图像数据
image = uint8(zeros(height, width, 3));
% 将图像数据写入文件
fileID = fopen('output.ppm', 'w');
fprintf(fileID, 'P6\n%d %d\n%d\n', width, height, maxColor);
fwrite(fileID, image, 'uint8');
fclose(fileID);
```
在这个示例中,我们首先定义了图像的宽度、高度和颜色深度。然后,我们创建了一个与图像大小相同的 uint8 类型的零矩阵,表示我们要写入的图像数据。
接下来,我们使用 fopen 函数打开一个名为 output.ppm 的文件,并使用 fprintf 函数向文件中写入 PPM 文件头信息。最后,我们使用 fwrite 函数将图像数据写入文件,并使用 fclose 函数关闭文件。
请注意,这个示例中的图像数据是全黑的,如果你需要写入自己的图像数据,需要将 image 矩阵替换为你自己的数据。
相关问题
matlab通过fopen实现写入PPM图像P3 和P6的功能
可以使用Matlab中的`fopen`和`fwrite`函数来实现写入PPM图像的功能。
以写入P3格式的PPM图像为例,代码如下:
```matlab
% 生成图像数据
img = uint8(rand(100, 100, 3) * 255);
% 打开文件
file = fopen('test.ppm', 'w');
% 写入头部信息
fprintf(file, 'P3\n');
fprintf(file, '%d %d\n', size(img, 2), size(img, 1));
fprintf(file, '255\n');
% 写入像素信息
for i = 1:size(img, 1)
for j = 1:size(img, 2)
fprintf(file, '%d %d %d\n', img(i, j, 1), img(i, j, 2), img(i, j, 3));
end
end
% 关闭文件
fclose(file);
```
其中,第一行代码生成一个随机的RGB图像,大小为100*100。第3-5行代码写入PPM头部信息。第7-11行代码遍历图像像素,将其写入文件中。最后,关闭文件。
如果要写入P6格式的PPM图像,只需要将第3行改为`fprintf(file, 'P6\n');`,并使用`fwrite`函数写入二进制数据即可。代码如下:
```matlab
% 生成图像数据
img = uint8(rand(100, 100, 3) * 255);
% 打开文件
file = fopen('test.ppm', 'wb');
% 写入头部信息
fprintf(file, 'P6\n');
fprintf(file, '%d %d\n', size(img, 2), size(img, 1));
fprintf(file, '255\n');
% 写入像素信息
fwrite(file, img, 'uint8');
% 关闭文件
fclose(file);
```
其中,第7行使用`fwrite`函数将图像数据写入文件中。
matlab通过fopen实现读取和写入PPM图像的功能
可以使用MATLAB中的fopen、fread、fwrite和fclose函数实现读取和写入PPM图像的功能。以下是示例代码:
读取PPM图像:
```matlab
% 打开PPM文件
fid = fopen('example.ppm', 'r');
% 读取文件头信息
header = fgets(fid);
% 读取图像宽度和高度信息
size = fscanf(fid, '%d %d', [1 2]);
% 读取最大像素值信息
maxval = fscanf(fid, '%d', 1);
% 读取图像数据
img = fread(fid, [size(1) size(2)], 'uint8');
% 关闭文件
fclose(fid);
```
写入PPM图像:
```matlab
% 打开PPM文件
fid = fopen('example_out.ppm', 'w');
% 写入文件头信息
fprintf(fid, 'P6\n%d %d\n%d\n', size(1), size(2), maxval);
% 写入图像数据
fwrite(fid, img, 'uint8');
% 关闭文件
fclose(fid);
```
需要注意的是,读取PPM图像时需要按照PPM文件格式的顺序依次读取文件头信息、图像宽度和高度信息、最大像素值信息和图像数据。写入PPM图像时需要按照PPM文件格式的顺序依次写入文件头信息、图像宽度和高度信息、最大像素值信息和图像数据。
相关推荐
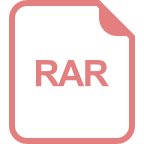
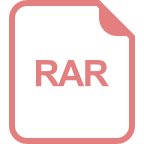









