matlab通过fopen实现一个函数读取和写入PPM和PGM图像的功能
时间: 2024-02-25 18:58:25 浏览: 21
可以使用以下函数读取和写入PPM和PGM图像:
```matlab
function img = readImage(filename)
% Read PPM or PGM image file
% Usage: img = readImage(filename)
% Input:
% filename - string, the image file name
% Output:
% img - a 3D array, the image data
fid = fopen(filename, 'r');
if fid < 0
error('Unable to open file');
end
% Read header
header = fgetl(fid);
if strcmp(header, 'P6')
color = true;
elseif strcmp(header, 'P5')
color = false;
else
error('Invalid image format');
end
line = fgetl(fid);
while line(1) == '#'
line = fgetl(fid);
end
[width, height] = sscanf(line, '%d %d');
maxval = fscanf(fid, '%d', 1);
% Read image data
if color
img = uint8(zeros(height, width, 3));
data = fread(fid, [3, width*height], 'uint8');
img(:, :, 1) = reshape(data(1, :), [width, height])';
img(:, :, 2) = reshape(data(2, :), [width, height])';
img(:, :, 3) = reshape(data(3, :), [width, height])';
else
img = uint8(zeros(height, width));
data = fread(fid, [1, width*height], 'uint8');
img(:, :) = reshape(data, [width, height])';
end
fclose(fid);
end
function writeImage(filename, img)
% Write PPM or PGM image file
% Usage: writeImage(filename, img)
% Input:
% filename - string, the image file name
% img - a 3D array, the image data
if size(img, 3) == 3
color = true;
elseif size(img, 3) == 1
color = false;
else
error('Invalid image format');
end
if color
header = 'P6';
else
header = 'P5';
end
fid = fopen(filename, 'w');
if fid < 0
error('Unable to open file');
end
fprintf(fid, '%s\n%d %d\n%d\n', header, size(img, 2), size(img, 1), 255);
if color
fwrite(fid, permute(img, [3, 1, 2]), 'uint8');
else
fwrite(fid, img', 'uint8');
end
fclose(fid);
end
```
使用示例:
```matlab
% Read PPM image
img_ppm = readImage('image.ppm');
% Write PGM image
img_pgm = rgb2gray(img_ppm);
writeImage('image.pgm', img_pgm);
```
相关推荐
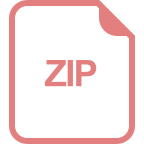
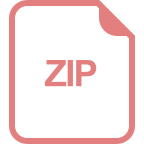
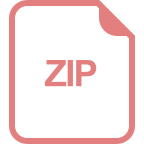














