matlab实现读取和写入PPM和PGM图像
时间: 2024-01-29 13:04:29 浏览: 35
读取PPM图像的代码示例:
```matlab
% 打开文件
fid = fopen('image.ppm', 'r');
% 读取文件头
tline = fgetl(fid);
% 检查是否为PPM格式
if ~strcmp(tline, 'P3')
error('不是PPM格式图像');
end
% 读取图像宽度、高度、像素最大值
tline = fgetl(fid);
width_height = sscanf(tline, '%d');
width = width_height(1);
height = width_height(2);
max_color = sscanf(fgetl(fid), '%d', 1);
% 读取像素值
pixel_data = fscanf(fid, '%d', [3, width * height]);
% 关闭文件
fclose(fid);
% 转换成RGB图像
image_data = reshape(pixel_data, [3, width, height]);
image_data = permute(image_data, [3, 2, 1]);
image_data = uint8(image_data);
```
写入PPM图像的代码示例:
```matlab
% 打开文件
fid = fopen('image.ppm', 'w');
% 写入文件头
fprintf(fid, 'P3\n%d %d\n%d\n', width, height, max_color);
% 写入像素值
pixel_data = permute(image_data, [3, 2, 1]);
pixel_data = reshape(pixel_data, [3, width * height]);
fprintf(fid, '%d %d %d\n', pixel_data);
% 关闭文件
fclose(fid);
```
读取PGM图像的代码示例:
```matlab
% 打开文件
fid = fopen('image.pgm', 'r');
% 读取文件头
tline = fgetl(fid);
% 检查是否为PGM格式
if ~strcmp(tline, 'P2')
error('不是PGM格式图像');
end
% 读取图像宽度、高度、像素最大值
tline = fgetl(fid);
width_height = sscanf(tline, '%d');
width = width_height(1);
height = width_height(2);
max_gray = sscanf(fgetl(fid), '%d', 1);
% 读取像素值
pixel_data = fscanf(fid, '%d', [1, width * height]);
% 关闭文件
fclose(fid);
% 转换成灰度图像
image_data = reshape(pixel_data, [width, height]);
image_data = image_data';
image_data = uint8(image_data);
```
写入PGM图像的代码示例:
```matlab
% 打开文件
fid = fopen('image.pgm', 'w');
% 写入文件头
fprintf(fid, 'P2\n%d %d\n%d\n', width, height, max_gray);
% 写入像素值
pixel_data = reshape(image_data', [1, width * height]);
fprintf(fid, '%d\n', pixel_data);
% 关闭文件
fclose(fid);
```
相关推荐













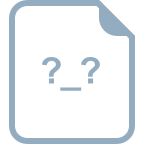