matlab通过fopen实现读取和写入PPM图像的功能
时间: 2023-12-30 15:06:04 浏览: 50
可以使用MATLAB中的fopen、fread、fwrite和fclose函数实现读取和写入PPM图像的功能。以下是示例代码:
读取PPM图像:
```matlab
% 打开PPM文件
fid = fopen('example.ppm', 'r');
% 读取文件头信息
header = fgets(fid);
% 读取图像宽度和高度信息
size = fscanf(fid, '%d %d', [1 2]);
% 读取最大像素值信息
maxval = fscanf(fid, '%d', 1);
% 读取图像数据
img = fread(fid, [size(1) size(2)], 'uint8');
% 关闭文件
fclose(fid);
```
写入PPM图像:
```matlab
% 打开PPM文件
fid = fopen('example_out.ppm', 'w');
% 写入文件头信息
fprintf(fid, 'P6\n%d %d\n%d\n', size(1), size(2), maxval);
% 写入图像数据
fwrite(fid, img, 'uint8');
% 关闭文件
fclose(fid);
```
需要注意的是,读取PPM图像时需要按照PPM文件格式的顺序依次读取文件头信息、图像宽度和高度信息、最大像素值信息和图像数据。写入PPM图像时需要按照PPM文件格式的顺序依次写入文件头信息、图像宽度和高度信息、最大像素值信息和图像数据。
相关问题
matlab通过fopen实现读取和写入PPM图像P3 和P6的功能
以下是通过`fopen`在MATLAB中实现读取和写入PPM图像P3和P6的示例代码:
读取P3格式的PPM图像:
```matlab
fid = fopen('image.ppm', 'r');
if fid == -1
error('Unable to open file.');
end
% Read file header
fgetl(fid); % Skip magic number
fgetl(fid); % Skip comment
dims = sscanf(fgetl(fid), '%d %d', [1, 2]);
max_val = sscanf(fgetl(fid), '%d', 1);
% Read image data
data = fscanf(fid, '%d %d %d', [3, dims(1)*dims(2)]);
data = data';
% Close the file
fclose(fid);
% Reshape the data into a 3D matrix
img = reshape(data, [dims(2), dims(1), 3]);
img = uint8(img);
```
写入P3格式的PPM图像:
```matlab
img = imread('image.png');
img = double(img);
% Open file for writing
fid = fopen('image.ppm', 'w');
if fid == -1
error('Unable to open file.');
end
% Write file header
fprintf(fid, 'P3\n');
fprintf(fid, '# Created by MATLAB\n');
fprintf(fid, '%d %d\n', size(img, 2), size(img, 1));
fprintf(fid, '%d\n', 255);
% Write image data
for i = 1:size(img, 1)
for j = 1:size(img, 2)
fprintf(fid, '%d %d %d ', img(i, j, 1), img(i, j, 2), img(i, j, 3));
end
fprintf(fid, '\n');
end
% Close the file
fclose(fid);
```
读取P6格式的PPM图像:
```matlab
fid = fopen('image.ppm', 'rb');
if fid == -1
error('Unable to open file.');
end
% Read file header
fgetl(fid); % Skip magic number
fgetl(fid); % Skip comment
dims = sscanf(fgetl(fid), '%d %d', [1, 2]);
max_val = sscanf(fgetl(fid), '%d', 1);
% Read image data
data = fread(fid, [dims(1)*3, dims(2)], 'uint8');
data = reshape(data, [3, dims(2), dims(1)]);
data = permute(data, [3, 2, 1]);
% Close the file
fclose(fid);
% Convert to uint8
img = uint8(data);
```
写入P6格式的PPM图像:
```matlab
img = imread('image.png');
% Open file for writing
fid = fopen('image.ppm', 'wb');
if fid == -1
error('Unable to open file.');
end
% Write file header
fprintf(fid, 'P6\n');
fprintf(fid, '# Created by MATLAB\n');
fprintf(fid, '%d %d\n', size(img, 2), size(img, 1));
fprintf(fid, '%d\n', 255);
% Write image data
fwrite(fid, img, 'uint8');
% Close the file
fclose(fid);
```
请注意,这些示例代码仅适用于基本的PPM图像格式,您可能需要根据您的具体需求进行修改。此外,该代码仅支持8位图像数据。如果您的图像数据是16位或32位,请相应地进行修改。
matlab通过fopen实现读取和写入PPM和PGM图像的功能
可以使用Matlab的`fopen`、`fread`和`fwrite`函数来读取和写入PPM和PGM图像。下面是一个示例,展示如何使用这些函数读取和写入PPM图像:
读取PPM图像:
```matlab
fid = fopen('image.ppm', 'r');
header = fgets(fid);
dim = fscanf(fid, '%d %d', [1,2]);
maxval = fscanf(fid, '%d', 1);
data = fread(fid, [dim(1), dim(2)*3], 'uint8');
fclose(fid);
% 将数据转换为RGB图像
img = zeros(dim(1), dim(2), 3, 'uint8');
img(:,:,1) = data(:,1:3:end);
img(:,:,2) = data(:,2:3:end);
img(:,:,3) = data(:,3:3:end);
```
写入PPM图像:
```matlab
fid = fopen('output.ppm', 'w');
fprintf(fid, 'P6\n%d %d\n%d\n', size(img,2), size(img,1), 255);
data = reshape(img, [], 3);
fwrite(fid, data', 'uint8');
fclose(fid);
```
其中,`data`是一个大小为`[height, width*3]`的矩阵,它包含PPM图像的RGB数据。在读取PPM图像时,我们将RGB数据存储在`data`矩阵中,然后将其转换为Matlab的RGB图像格式。在写入PPM图像时,我们将Matlab的RGB图像格式转换为`data`矩阵,并将其写入文件。
同样的方式,可以用以下代码读取和写入PGM图像:
读取PGM图像:
```matlab
fid = fopen('image.pgm', 'r');
header = fgets(fid);
dim = fscanf(fid, '%d %d', [1,2]);
maxval = fscanf(fid, '%d', 1);
data = fread(fid, [dim(1), dim(2)], 'uint8');
fclose(fid);
% 将数据转换为灰度图像
img = data;
```
写入PGM图像:
```matlab
fid = fopen('output.pgm', 'w');
fprintf(fid, 'P5\n%d %d\n%d\n', size(img,2), size(img,1), 255);
fwrite(fid, img', 'uint8');
fclose(fid);
```
在这个例子中,`data`是一个大小为`[height, width]`的矩阵,它包含PGM图像的灰度数据。在读取PGM图像时,我们将灰度数据存储在`data`矩阵中,然后将其转换为Matlab的灰度图像格式。在写入PGM图像时,我们将Matlab的灰度图像格式直接写入文件。
相关推荐
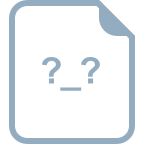
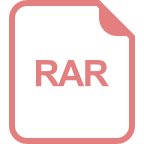
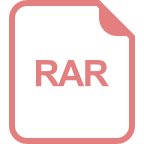







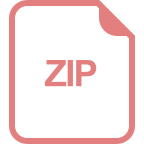
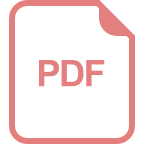
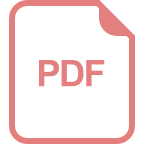
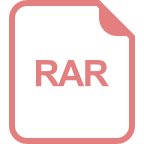
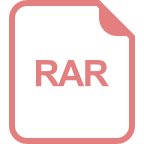
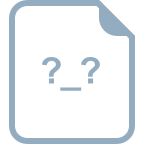