(transform, _) = cv2.findTransformECC(prev_gray, curr_gray, transform, motion_model, criteria) TypeError: findTransformECC() missing required argument 'inputMask' (pos 6)
时间: 2024-01-30 16:02:19 浏览: 25
这个错误通常是因为你使用的 OpenCV 版本较旧,缺少了一个必需的参数。在旧版本的 `cv2.findTransformECC()` 中,确实缺少了第六个参数 `inputMask`,但在较新的版本中已经添加了这个参数。
要解决这个问题,请尝试更新你的 OpenCV 版本。如果无法更新,则可以尝试更改代码以使用旧版本的 `cv2.findTransformECC()`,并省略 `inputMask` 参数。或者,你可以尝试手动安装最新版本的 OpenCV。下面是一些安装最新版本的 OpenCV 的方法:
使用 pip 安装:
```
pip install opencv-python-headless
```
使用 Anaconda 安装:
```
conda install -c conda-forge opencv
```
使用源代码编译安装:
```
git clone https://github.com/opencv/opencv.git
cd opencv
mkdir build
cd build
cmake ..
make
sudo make install
```
请注意,编译和安装可能需要一些时间,并且需要安装一些依赖项。
相关问题
对下面代码每一步含义进行注释 def convert_to_doubly_linked_list(self): if not self.root: return None def convert(root): if not root.left and not root.right: return ListNode(root.val) if not root.left: right_head = convert(root.right) right_tail = right_head while right_tail.next: right_tail = right_tail.next cur_node = ListNode(root.val, None, right_head) right_head.prev = cur_node return cur_node if not root.right: left_tail = convert(root.left) left_head = left_tail while left_head.prev: left_head = left_head.prev cur_node = ListNode(root.val, left_tail, None) left_tail.next = cur_node return cur_node left_tail = convert(root.left) right_head = convert(root.right) left_head = left_tail while left_head.prev: left_head = left_head.prev right_tail = right_head while right_tail.next: right_tail = right_tail.next cur_node = ListNode(root.val, left_tail, right_head) left_tail.next = cur_node right_head.prev = cur_node return left_head return convert(self.root) def inorder_traversal(self, root): if not root: return self.inorder_traversal(root.left) print(root.val, end=' ') self.inorder_traversal(root.right) def print_bst(self): self.inorder_traversal(self.root) print() def traverse_doubly_linked_list(self, head): cur_node = head while cur_node: print(cur_node.val, end=' ') cur_node = cur_node.next print() def reverse_traverse_doubly_linked_list(self, head): cur_node = head while cur_node.next: cur_node = cur_node.next while cur_node: print(cur_node.val, end=' ') cur_node = cur_node.prev print()
这段代码是一个二叉搜索树(BST)转化为双向链表的函数,同时提供了打印BST和遍历双向链表的函数。
- `def convert_to_doubly_linked_list(self):`:定义一个将BST转化为双向链表的函数。
- `if not self.root:`:如果BST为空,则返回None。
- `def convert(root):`:定义一个递归函数,将BST转化为双向链表。
- `if not root.left and not root.right:`:如果该节点没有左右子树,返回一个只包含该节点值的ListNode。
- `if not root.left:`:如果该节点没有左子树,将右子树转化为双向链表,并将节点值作为新的头结点,返回该节点。
- `if not root.right:`:如果该节点没有右子树,将左子树转化为双向链表,并将节点值作为新的尾结点,返回该节点。
- `left_tail = convert(root.left)`:将左子树转化为双向链表,并返回左子树的尾结点。
- `right_head = convert(root.right)`:将右子树转化为双向链表,并返回右子树的头结点。
- `left_head = left_tail`:将左子树的头结点设置为左子树的尾结点。
- `while left_head.prev:`:找到左子树双向链表的头结点。
- `right_tail = right_head`:将右子树的尾结点设置为右子树的头结点。
- `while right_tail.next:`:找到右子树双向链表的尾结点。
- `cur_node = ListNode(root.val, left_tail, right_head)`:创建一个新的节点,值为当前节点值,左指针指向左子树双向链表的尾结点,右指针指向右子树双向链表的头结点。
- `left_tail.next = cur_node`:将左子树双向链表的尾结点的右指针指向新节点。
- `right_head.prev = cur_node`:将右子树双向链表的头结点的左指针指向新节点。
- `return left_head`:返回双向链表的头结点。
- `return convert(self.root)`:调用递归函数convert并返回结果。
- `def inorder_traversal(self, root):`:定义一个中序遍历BST的函数。
- `if not root:`:如果该节点为空,则返回。
- `self.inorder_traversal(root.left)`:递归遍历左子树。
- `print(root.val, end=' ')`:输出当前节点的值。
- `self.inorder_traversal(root.right)`:递归遍历右子树。
- `def print_bst(self):`:定义一个打印BST的函数。
- `self.inorder_traversal(self.root)`:调用中序遍历函数遍历BST。
- `print()`:输出一个空行。
- `def traverse_doubly_linked_list(self, head):`:定义一个遍历双向链表的函数。
- `cur_node = head`:将当前节点指向链表的头结点。
- `while cur_node:`:遍历整个链表,直到当前节点为空。
- `print(cur_node.val, end=' ')`:输出当前节点的值。
- `cur_node = cur_node.next`:将当前节点指向下一个节点。
- `print()`:输出一个空行。
- `def reverse_traverse_doubly_linked_list(self, head):`:定义一个逆序遍历双向链表的函数。
- `cur_node = head`:将当前节点指向链表的头结点。
- `while cur_node.next:`:找到链表的尾结点。
- `cur_node = cur_node.next`:将当前节点指向下一个节点。
- `while cur_node:`:逆序遍历整个链表,直到当前节点为空。
- `print(cur_node.val, end=' ')`:输出当前节点的值。
- `cur_node = cur_node.prev`:将当前节点指向上一个节点。
- `print()`:输出一个空行。
cv2.cvtColor(prev_frame, cv2.COLOR_BGR2GRAY)
cv2.cvtColor(prev_frame, cv2.COLOR_BGR2GRAY)是一个OpenCV函数,用于将彩色图像转换为灰度图像。其中prev_frame是输入的彩色图像,cv2.COLOR_BGR2GRAY是转换的颜色空间,表示将BGR彩色空间转换为灰度空间。这个函数的返回值是转换后的灰度图像。
相关推荐
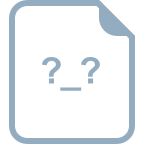
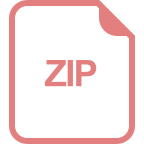
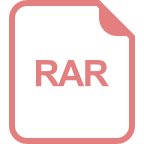












