Eigen判断矩阵是否可逆
时间: 2023-07-26 10:29:30 浏览: 763
对于一个n×n的矩阵A,如果其行列式det(A)不等于0,那么A是可逆的,也就是说它存在逆矩阵。因此,可以使用Eigen库中的determinant()函数来判断矩阵是否可逆。示例代码如下:
```
#include <iostream>
#include <Eigen/Dense>
using namespace Eigen;
int main()
{
Matrix2f A;
A << 1, 2,
3, 4;
std::cout << "A:\n" << A << std::endl;
float det_A = A.determinant();
if (det_A != 0)
{
std::cout << "A is invertible." << std::endl;
}
else
{
std::cout << "A is not invertible." << std::endl;
}
return 0;
}
```
其中,Matrix2f表示一个2x2的浮点矩阵,<<运算符用于初始化矩阵A,determinant()函数用于求矩阵A的行列式。如果行列式不等于0,则矩阵A是可逆的。
相关问题
c++一个复数矩阵可逆,但是执行inverse()时,结果却为-nan(ind)
一个复数矩阵可逆,但是执行inverse()方法时结果为-nan(ind)的原因可能是因为矩阵元素中存在非常小的数或者非数(NaN)。
在实际的计算过程中,可能会出现数值误差,导致矩阵的元素中存在非常小的数或者非数(NaN)。这些数值误差可能会影响矩阵的计算结果,导致计算结果不准确。
为了解决这个问题,可以使用 Eigen 库中的 MatrixBase::setIdentity() 方法来设置单位矩阵,然后使用 MatrixBase::lu() 方法将矩阵分解为 LU 分解,最后使用 MatrixBase::solve() 方法求解线性方程组。这种方法可以避免直接计算逆矩阵时出现的数值误差。
以下是一个示例代码:
```c++
#include <iostream>
#include <Eigen/Dense>
int main()
{
// 定义一个3x3的复数矩阵
Eigen::MatrixXcd A(3,3);
A << std::complex<double>(1,2), std::complex<double>(3,4), std::complex<double>(5,6),
std::complex<double>(7,8), std::complex<double>(9,10), std::complex<double>(11,12),
std::complex<double>(13,14), std::complex<double>(15,16), std::complex<double>(17,18);
// 判断矩阵是否可逆
if(A.determinant() == std::complex<double>(0))
{
std::cout << "Matrix is not invertible." << std::endl;
return 0;
}
// 设置单位矩阵
Eigen::MatrixXcd I = Eigen::MatrixXcd::Identity(3,3);
// 将矩阵分解为 LU 分解
Eigen::PartialPivLU<Eigen::MatrixXcd> lu(A);
// 解线性方程组
Eigen::MatrixXcd A_inv = lu.solve(I);
// 输出逆矩阵
std::cout << "Inverse matrix:" << std::endl << A_inv << std::endl;
return 0;
}
```
这个示例代码使用了 LU 分解和线性方程组求解方法,避免了直接计算逆矩阵时出现的数值误差。如果矩阵可逆,程序会输出逆矩阵的值。
用C++代码写一个矩阵的加减乘除、逆矩阵
在C++中,我们可以使用二维数组来表示矩阵,并提供相应的函数来执行基本的矩阵运算,如加法、减法、乘法和求逆(如果矩阵是方阵且可逆)。下面是一个简单的示例,展示了如何创建矩阵类和实现基本操作:
```cpp
#include <iostream>
#include <vector>
class Matrix {
public:
// 初始化矩阵
Matrix(int rows, int cols, double values = 0) : m_rows(rows), m_cols(cols) {
data.resize(rows * cols);
for (int i = 0; i < rows * cols; ++i)
data[i] = values;
}
// 获取矩阵尺寸
int getRows() const { return m_rows; }
int getCols() const { return m_cols; }
// 矩阵加法
Matrix operator+(const Matrix& other) {
if (m_rows != other.m_rows || m_cols != other.m_cols)
throw std::invalid_argument("Matrices must have the same dimensions to add");
Matrix result(*this);
for (size_t i = 0; i < m_rows * m_cols; ++i)
result.data[i] += other.data[i];
return result;
}
// 矩阵减法
Matrix operator-(const Matrix& other) {
if (m_rows != other.m_rows || m_cols != other.m_cols)
throw std::invalid_argument("Matrices must have the same dimensions to subtract");
Matrix result(*this);
for (size_t i = 0; i < m_rows * m_cols; ++i)
result.data[i] -= other.data[i];
return result;
}
// 矩阵乘法,仅适用于方阵
friend Matrix operator*(const Matrix& left, const Matrix& right) {
if (left.getCols() != right.getRows())
throw std::invalid_argument("Number of columns in first matrix must equal number of rows in second matrix for multiplication");
Matrix result(left.getRows(), right.getCols());
for (int i = 0; i < left.getRows(); ++i) {
for (int j = 0; j < right.getCols(); ++j) {
double sum = 0;
for (int k = 0; k < left.getCols(); ++k)
sum += left.data[i * left.getCols() + k] * right.data[k * right.getCols() + j];
result.data[i * right.getCols() + j] = sum;
}
}
return result;
}
private:
int m_rows, m_cols;
std::vector<double> data;
};
// 只有当矩阵是方阵且行列式非零时才可求逆
bool isSquareAndInvertible(const Matrix& mat) {
return mat.getRows() == mat.getCols() && !isZeroDeterminant(mat);
}
// 求矩阵行列式
double determinant(const Matrix& mat) {
// 实现行展开等算法计算行列式,这里简化只处理2x2矩阵
if (mat.getRows() == 2 && mat.getCols() == 2) {
return mat.data[0] * mat.data[3] - mat.data[1] * mat.data[2];
} else {
throw std::runtime_error("Determinant calculation not supported for matrices larger than 2x2");
}
}
// 判断方阵是否可逆
bool isZeroDeterminant(const Matrix& mat) {
return determinant(mat) == 0;
}
// 仅适用于方阵的逆矩阵
Matrix inverse(const Matrix& mat) {
if (!isSquareAndInvertible(mat))
throw std::runtime_error("Cannot compute inverse of singular matrix");
// 使用高斯消元或其他方法求逆,这里是简化的例子
// 仅用于展示思路,实际实现需要更复杂的算法
Matrix inv_mat(mat.getRows(), mat.getCols());
for (int i = 0; i < mat.getRows(); ++i) {
for (int j = i; j < mat.getCols(); ++j) {
double factor = mat.data[j * mat.getCols() + i] / determinant(mat);
inv_mat.data[i * mat.getCols() + j] = factor;
for (int k = 0; k < mat.getCols(); ++k)
inv_mat.data[i * mat.getCols() + k] -= factor * mat.data[j * mat.getCols() + k];
}
}
return inv_mat;
}
int main() {
Matrix a(2, 2, {1, 2, 3, 4});
Matrix b(2, 2, {5, 6, 7, 8});
Matrix c = a + b;
Matrix d = a - b;
Matrix e = a * b;
if (a.getCols() == b.getRows()) {
Matrix f = a * inverse(b);
// 打印结果
std::cout << "Matrix addition: \n" << c << "\n";
std::cout << "Matrix subtraction: \n" << d << "\n";
std::cout << "Matrix multiplication: \n" << e << "\n";
if (isSquareAndInvertible(a)) {
std::cout << "Inverse of A: \n" << f << "\n";
} else {
std::cout << "A is not invertible.\n";
}
} else {
std::cerr << "Can't multiply matrices without matching dimensions\n";
}
return 0;
}
```
请注意,这个示例中的代码并不是完整的矩阵操作库,而是为了演示基本概念而设计的,特别是矩阵乘法和逆矩阵的部分。对于实际项目,你可能会选择使用成熟的数学库,如Eigen或Boost.Multiprecision。
阅读全文
相关推荐
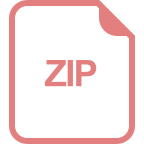
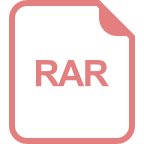
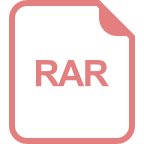
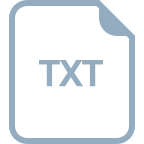


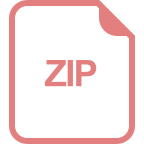