tensorflow lstm模型
时间: 2023-09-03 11:08:17 浏览: 54
LSTM(长短期记忆)是一种循环神经网络(RNN)的变体,它可以处理具有长期依赖关系的序列数据。TensorFlow是一个流行的机器学习框架,支持LSTM模型的实现。
在TensorFlow中,可以使用以下步骤来构建LSTM模型:
1. 导入必要的库和模块:TensorFlow、NumPy等。
2. 准备数据集:将数据集进行预处理和分割,以便用于模型的训练和测试。
3. 构建LSTM模型:使用TensorFlow的API创建LSTM模型,包括定义输入层、隐藏层、输出层、损失函数和优化器等。
4. 训练LSTM模型:使用TensorFlow的API训练模型,调整模型参数以最小化损失函数。
5. 测试LSTM模型:使用TensorFlow的API测试模型,评估模型性能并预测新数据。
以下是一个简单的TensorFlow LSTM模型的示例代码:
```python
import tensorflow as tf
import numpy as np
# 准备数据集
train_data = [1.0, 2.0, 3.0, 4.0, 5.0]
train_labels = [2.0, 4.0, 6.0, 8.0, 10.0]
# 定义模型参数
num_input = 1
num_steps = 1
num_hidden = 10
num_output = 1
# 定义输入和输出
inputs = tf.placeholder(tf.float32, [None, num_steps, num_input])
labels = tf.placeholder(tf.float32, [None, num_output])
# 定义LSTM模型
lstm_cell = tf.contrib.rnn.BasicLSTMCell(num_hidden, forget_bias=1.0)
outputs, states = tf.nn.dynamic_rnn(lstm_cell, inputs, dtype=tf.float32)
outputs = tf.transpose(outputs, [1, 0, 2])
last_output = tf.gather(outputs, int(outputs.get_shape()[0]) - 1)
weights = tf.Variable(tf.truncated_normal([num_hidden, num_output]))
bias = tf.Variable(tf.constant(0.1, shape=[num_output]))
predictions = tf.matmul(last_output, weights) + bias
# 定义损失函数和优化器
loss = tf.reduce_mean(tf.square(predictions - labels))
optimizer = tf.train.AdamOptimizer(learning_rate=0.01).minimize(loss)
# 训练模型
num_epochs = 1000
batch_size = 1
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(num_epochs):
epoch_loss = 0
for i in range(len(train_data)):
batch_inputs = np.array(train_data[i]).reshape([-1, num_steps, num_input])
batch_labels = np.array(train_labels[i]).reshape([-1, num_output])
_, batch_loss = sess.run([optimizer, loss], feed_dict={inputs: batch_inputs, labels: batch_labels})
epoch_loss += batch_loss
print('Epoch', epoch+1, 'loss:', epoch_loss)
# 测试模型
test_data = [6.0, 7.0, 8.0, 9.0, 10.0]
test_labels = [12.0, 14.0, 16.0, 18.0, 20.0]
test_inputs = np.array(test_data).reshape([-1, num_steps, num_input])
test_predictions = sess.run(predictions, feed_dict={inputs: test_inputs})
print('Test predictions:', test_predictions)
```
在上述代码中,我们使用TensorFlow的API来定义LSTM模型,包括输入层、隐藏层、输出层、损失函数和优化器等。然后,我们使用训练数据训练模型,并使用测试数据测试模型。最终,我们输出测试数据的预测结果。
相关推荐
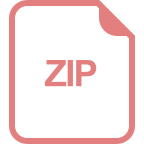
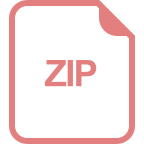
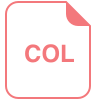
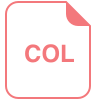
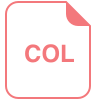

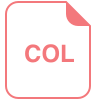









