请帮我用pytorch写出SparseAttention的代码
时间: 2024-02-15 12:03:17 浏览: 18
当然可以,下面是一个简单的SparseAttention实现代码:
```python
import torch
import torch.nn.functional as F
class SparseAttention(torch.nn.Module):
def __init__(self, num_heads, head_dim, dropout=0.0):
super(SparseAttention, self).__init__()
self.num_heads = num_heads
self.head_dim = head_dim
self.dropout = dropout
self.scale = head_dim ** -0.5
self.qkv_proj = torch.nn.Linear(head_dim * num_heads, head_dim * 3 * num_heads, bias=False)
self.out_proj = torch.nn.Linear(head_dim * num_heads, head_dim * num_heads, bias=False)
def forward(self, query, key, value, attention_mask=None):
batch_size, seq_len, hidden_size = query.shape
num_heads = self.num_heads
head_dim = self.head_dim
# Project query, key, value
qkv = self.qkv_proj(torch.cat((query, key, value), dim=-1))
q, k, v = torch.chunk(qkv, 3, dim=-1)
q = q.view(batch_size, seq_len, num_heads, head_dim)
k = k.view(batch_size, seq_len, num_heads, head_dim)
v = v.view(batch_size, seq_len, num_heads, head_dim)
# Compute attention scores
attention_scores = q @ k.transpose(-1, -2)
attention_scores *= self.scale
# Apply attention mask (if provided)
if attention_mask is not None:
attention_scores.masked_fill_(~attention_mask.unsqueeze(1), -1e9)
# Compute attention weights and apply dropout
attention_weights = F.softmax(attention_scores, dim=-1)
attention_weights = F.dropout(attention_weights, p=self.dropout, training=self.training)
# Compute attention output
attention_output = attention_weights @ v
# Reshape and project back to hidden size
attention_output = attention_output.view(batch_size, seq_len, num_heads * head_dim)
attention_output = self.out_proj(attention_output)
return attention_output
```
在这个实现中,我们使用了 `torch.nn.Linear` 来处理所有的线性变换,使用了 `torch.chunk` 来将输出拆成多个头,使用了 `torch.matmul` 来计算注意力分数,使用了 `F.softmax` 来计算注意力权重,使用了 `F.dropout` 来进行dropout,最后使用了 `torch.matmul` 将注意力权重应用于value上。
注意,这个实现并没有对注意力矩阵进行稀疏化处理,但是可以通过一些技巧来实现。
相关推荐
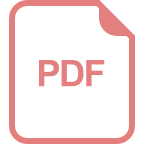
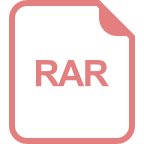





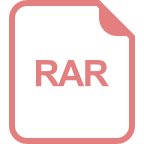
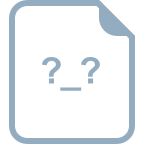
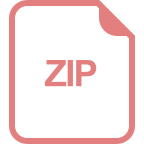
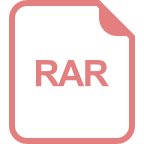
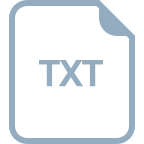
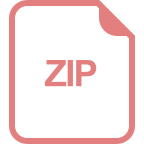