pyqt5实现人脸识别
时间: 2023-08-29 08:12:00 浏览: 148
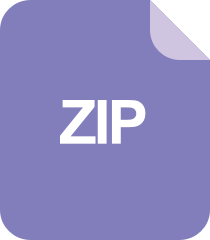
Python-基于PyQt5实现的人脸采集系统

要在PyQt5中实现人脸识别,您需要使用OpenCV库来完成人脸检测和识别。以下是一个简单的示例,展示了如何使用PyQt5和OpenCV库来实现人脸识别:
```python
import cv2
import sys
from PyQt5.QtCore import QTimer
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.faceCascade = cv2.CascadeClassifier("haarcascade_frontalface_default.xml") # 加载人脸分类器
self.videoCapture = cv2.VideoCapture(0) # 打开摄像头
self.videoCapture.set(cv2.CAP_PROP_FRAME_WIDTH, 640) # 设置摄像头分辨率
self.videoCapture.set(cv2.CAP_PROP_FRAME_HEIGHT, 480)
self.timer = QTimer(self) # 创建一个定时器
self.timer.timeout.connect(self.update_frame) # 将定时器的timeout信号连接到update_frame槽
self.timer.start(1) # 开始定时器,每1ms更新一次
self.label = QLabel(self) # 创建一个标签用于显示视频帧
self.setCentralWidget(self.label) # 将标签设置为中心部件
self.setWindowTitle("Face Recognition") # 设置窗口标题
self.show() # 显示窗口
def update_frame(self):
ret, frame = self.videoCapture.read() # 读取视频帧
if ret:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # 将视频帧转换成灰度图像
faces = self.faceCascade.detectMultiScale(gray, scaleFactor=1.2, minNeighbors=5, minSize=(30, 30)) # 检测人脸
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2) # 在视频帧上绘制矩形框
image = QImage(frame, frame.shape[1], frame.shape[0], QImage.Format_RGB888) # 将视频帧转换成QImage格式
pixmap = QPixmap.fromImage(image) # 将QImage转换成QPixmap格式
self.label.setPixmap(pixmap) # 在标签上显示视频帧
if __name__ == '__main__':
app = QApplication(sys.argv)
mainWindow = MainWindow()
sys.exit(app.exec_())
```
在此示例中,我们首先加载了OpenCV的人脸分类器,然后打开了摄像头,并创建了一个定时器来定期读取视频帧并更新标签以显示视频帧。 在update_frame函数中,我们将视频帧转换为灰度图像,并使用人脸分类器检测人脸。 如果有人脸被检测到,我们将在视频帧上绘制一个矩形框,并将视频帧转换为QImage格式,然后转换为QPixmap格式,并在标签上显示视频帧。
阅读全文
相关推荐
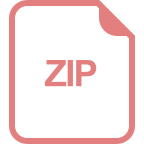
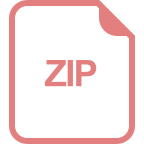

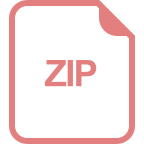
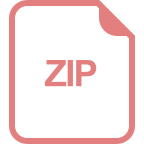
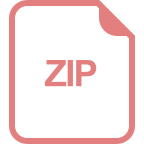
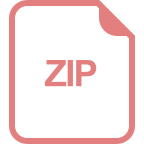
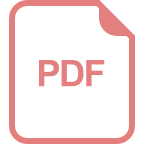
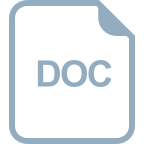
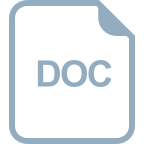
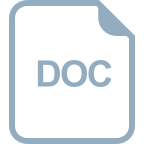



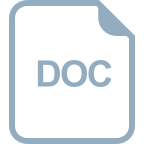
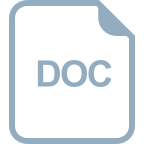

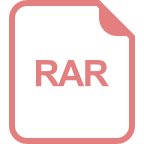