std::string myplus(const std::string& a, const std::string& b){ }
时间: 2023-07-08 13:09:24 浏览: 45
这是一个函数声明,它声明了一个名为myplus的函数,该函数接受两个const引用类型的std::string参数a和b,并且返回一个std::string类型的值。该函数的实现可能如下:
```cpp
std::string myplus(const std::string& a, const std::string& b) {
return a + b;
}
```
这个函数的作用是将两个字符串a和b拼接在一起,并返回拼接后的结果。
相关问题
const std::string& 类型参数给 std::string类型参数赋值
对于将 const std::string& 类型参数赋值给 std::string 类型参数,可以使用构造函数或赋值运算符来完成。下面是两种常见的方法:
1. 使用构造函数:可以通过将 const std::string& 参数传递给 std::string 的构造函数来创建一个新的 std::string 对象。例如:
```cpp
const std::string& constStr = "Hello";
std::string str(constStr); // 使用构造函数进行赋值
```
2. 使用赋值运算符:可以使用赋值运算符将 const std::string& 的值赋给 std::string 对象。例如:
```cpp
const std::string& constStr = "Hello";
std::string str;
str = constStr; // 使用赋值运算符进行赋值
```
无论使用哪种方法,都会将 const std::string& 类型参数的值复制到 std::string 类型参数中,从而完成赋值操作。
写出3组Plus函数的声明与实现,类型分别为int,double,string。 主函数如下,注意只需要实现Plus函数和包含相关的头文件。 // Function prototype //int myplus(int a, int b); //double myplus(double a, double b); //std::string myplus(const std::string& a, const std::string& b); int main() { int n; int a,b; double c,d; std::string str1,str2; std::cin>>n; while(n--) { std::cin >> a >> b ; std::cin >> c >> d; std::cin >> str1 >> str2; std::cout << myplus(a, b) << endl; std::cout << myplus(d, c) << endl; std::cout << myplus(str1, str2) << endl; } return 0; }
int类型的Plus函数声明与实现:
声明:int myplus(int a, int b);
实现:
```
int myplus(int a, int b) {
return a + b;
}
```
double类型的Plus函数声明与实现:
声明:double myplus(double a, double b);
实现:
```
double myplus(double a, double b) {
return a + b;
}
```
string类型的Plus函数声明与实现:
声明:std::string myplus(const std::string& a, const std::string& b);
实现:
```
std::string myplus(const std::string& a, const std::string& b) {
return a + b;
}
```
在主函数中包含相关的头文件,如下所示:
```
#include <iostream>
#include <string>
```
完整的程序如下所示:
```
#include <iostream>
#include <string>
// Function prototype
int myplus(int a, int b);
double myplus(double a, double b);
std::string myplus(const std::string& a, const std::string& b);
int main() {
int n;
int a,b;
double c,d;
std::string str1,str2;
std::cin>>n;
while(n--) {
std::cin >> a >> b ;
std::cin >> c >> d;
std::cin >> str1 >> str2;
std::cout << myplus(a, b) << std::endl;
std::cout << myplus(d, c) << std::endl;
std::cout << myplus(str1, str2) << std::endl;
}
return 0;
}
int myplus(int a, int b) {
return a + b;
}
double myplus(double a, double b) {
return a + b;
}
std::string myplus(const std::string& a, const std::string& b) {
return a + b;
}
```
相关推荐
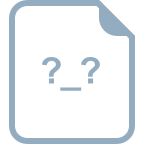
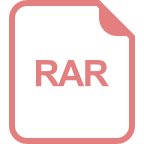
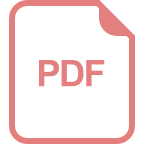












