Create the layout for your digital clock. The clock should have the following layout
时间: 2024-02-15 13:06:07 浏览: 101
Here is a possible layout for a digital clock:
```
_______________________
| 12:34:56 |
|_______________________|
```
In this layout, the clock is centered on the screen and displays the time in hours, minutes, and seconds separated by colons. The font and size of the numbers can be adjusted to fit the design of the clock. Additionally, the clock can be customized with different colors, backgrounds, and animations to enhance its visual appeal.
相关问题
Use java to create the layout for your digital clock. The clock should have the following layout: so: ● HH - displays the hours ● MM - displays the minutes ● SS - displays the seconds ● AM/PM - displays whether it is before (AM) or after mid-day (PM). The hours/minutes/seconds should be displayed in 36 point, italic serif.
Sure, I can help you with that. Here's a sample Java code to create a digital clock with the given layout:
```
import java.awt.*;
import javax.swing.*;
public class DigitalClock extends JFrame {
private JLabel hourLabel, minuteLabel, secondLabel, amPmLabel;
public DigitalClock() {
initUI();
}
private void initUI() {
Container container = getContentPane();
container.setLayout(new GridLayout(1, 4));
hourLabel = new JLabel("HH", SwingConstants.CENTER);
hourLabel.setFont(new Font("Serif", Font.ITALIC, 36));
container.add(hourLabel);
minuteLabel = new JLabel("MM", SwingConstants.CENTER);
minuteLabel.setFont(new Font("Serif", Font.ITALIC, 36));
container.add(minuteLabel);
secondLabel = new JLabel("SS", SwingConstants.CENTER);
secondLabel.setFont(new Font("Serif", Font.ITALIC, 36));
container.add(secondLabel);
amPmLabel = new JLabel("AM/PM", SwingConstants.CENTER);
amPmLabel.setFont(new Font("SansSerif", Font.PLAIN, 18));
container.add(amPmLabel);
setTitle("Digital Clock");
setDefaultCloseOperation(EXIT_ON_CLOSE);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public static void main(String[] args) {
DigitalClock clock = new DigitalClock();
while (true) {
String time = getTime();
String[] parts = time.split(":");
clock.hourLabel.setText(parts[0]);
clock.minuteLabel.setText(parts[1]);
clock.secondLabel.setText(parts[2]);
if (Integer.parseInt(parts[0]) < 12) {
clock.amPmLabel.setText("AM");
} else {
clock.amPmLabel.setText("PM");
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private static String getTime() {
return java.time.LocalTime.now().toString();
}
}
```
This code creates a `JFrame` with a `GridLayout` of 1 row and 4 columns. It then creates 4 `JLabel` components for each part of the clock, and sets their font and alignment. Finally, it updates the clock every second using a `while` loop and the `java.time.LocalTime` class to get the current time.
Note that this is just a sample code, and you may need to modify it to suit your specific needs.
Create a digital clock a) Create the layout for your digital clock. The clock should have the following layout: so: ● HH - displays the hours ● MM - displays the minutes ● SS - displays the seconds ● AM/PM - displays whether it is before (AM) or after mid-day (PM). The hours/minutes/seconds should be displayed in 36 point, italic serif. (Correct layout: 0.25P, Class extends JFrame: 0.25P, Class follows OOP principles: 0.25P, Global set-up in main method: 0.25P)1 b) Make the clock show the current time. For this you should use the Calendar class from java.util with: ● Calendar.getInstance() - create Calendar object for current date/time ● get(field) - return integer field from Calendar object, where field may be Calendar.HOUR, Calendar.MINUTE, Calendar.SECOND, Calendar.AM_PM, etc. ● the value returned from Calendar.AM_PM may be compared with the values Calendar.AM and Calendar.PM to determine if it is before or after mid-day Create a function that runs continuously and updates the display to always show the current time.
Sure, I can help you with that. Here's the code for creating a digital clock in Java:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.GridLayout;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class DigitalClock extends JFrame {
private JLabel hourLabel, minuteLabel, secondLabel, amPmLabel;
public DigitalClock() {
setTitle("Digital Clock");
setSize(300, 150);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridLayout(2, 2));
panel.setBackground(Color.BLACK);
hourLabel = new JLabel("", JLabel.CENTER);
hourLabel.setFont(new Font("Serif", Font.ITALIC, 36));
hourLabel.setForeground(Color.WHITE);
panel.add(hourLabel);
minuteLabel = new JLabel("", JLabel.CENTER);
minuteLabel.setFont(new Font("Serif", Font.ITALIC, 36));
minuteLabel.setForeground(Color.WHITE);
panel.add(minuteLabel);
secondLabel = new JLabel("", JLabel.CENTER);
secondLabel.setFont(new Font("Serif", Font.ITALIC, 36));
secondLabel.setForeground(Color.WHITE);
panel.add(secondLabel);
amPmLabel = new JLabel("", JLabel.CENTER);
amPmLabel.setFont(new Font("Serif", Font.ITALIC, 20));
amPmLabel.setForeground(Color.WHITE);
panel.add(amPmLabel);
add(panel);
setVisible(true);
}
public void updateTime() {
Calendar calendar = Calendar.getInstance();
SimpleDateFormat timeFormat = new SimpleDateFormat("hh");
int hour = Integer.parseInt(timeFormat.format(calendar.getTime()));
timeFormat = new SimpleDateFormat("mm");
int minute = Integer.parseInt(timeFormat.format(calendar.getTime()));
timeFormat = new SimpleDateFormat("ss");
int second = Integer.parseInt(timeFormat.format(calendar.getTime()));
timeFormat = new SimpleDateFormat("a");
String amPm = timeFormat.format(calendar.getTime());
hourLabel.setText(String.format("%02d", hour));
minuteLabel.setText(String.format("%02d", minute));
secondLabel.setText(String.format("%02d", second));
amPmLabel.setText(amPm);
}
public static void main(String[] args) {
DigitalClock clock = new DigitalClock();
while (true) {
clock.updateTime();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
Here's how the code works:
- The `DigitalClock` class extends `JFrame` and creates a window with a 2x2 grid with labels for the hours, minutes, seconds, and AM/PM.
- The `updateTime` method gets the current time using `Calendar.getInstance()` and formats it using `SimpleDateFormat`.
- The `main` method creates an instance of the `DigitalClock` class and runs a continuous loop that calls the `updateTime` method every second using `Thread.sleep(1000)`.
I hope this helps you create your digital clock!
阅读全文
相关推荐

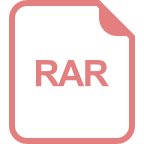
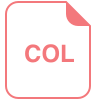
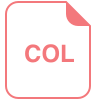
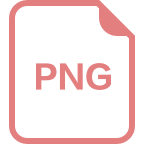
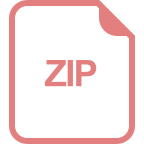
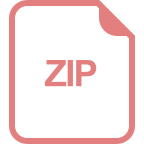
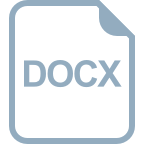