Create the layout for your digital clock. The clock should have the following layout: so: ● HH - displays the hours ● MM - displays the minutes ● SS - displays the seconds ● AM/PM - displays whether it is before (AM) or after mid-day (PM). The hours/minutes/seconds should be displayed in 36 point, italic serif. (Correct layout: 0.25P, Class extends JFrame: 0.25P, Class follows OOP principles: 0.25P, Global set-up in main method: 0.25P)1 b) Make the clock show the current time. For this you should use the Calendar class from java.util with: ● Calendar.getInstance() - create Calendar object for current date/time ● get(field) - return integer field from Calendar object, where field may be Calendar.HOUR, Calendar.MINUTE, Calendar.SECOND, Calendar.AM_PM, etc. ● the value returned from Calendar.AM_PM may be compared with the values Calendar.AM and Calendar.PM to determine if it is before or after mid-day Create a function that runs continuously and updates the display to always show the current time.
时间: 2024-02-07 19:04:05 浏览: 22
Sure, I can help you with that! Here is the layout code for the digital clock:
```java
import java.awt.Font;
import java.util.Calendar;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class DigitalClock extends JFrame {
private JLabel hourLabel;
private JLabel minuteLabel;
private JLabel secondLabel;
private JLabel amPmLabel;
public DigitalClock() {
setTitle("Digital Clock");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 150);
setLocationRelativeTo(null);
setResizable(false);
JPanel panel = new JPanel();
hourLabel = new JLabel("00");
minuteLabel = new JLabel("00");
secondLabel = new JLabel("00");
amPmLabel = new JLabel("AM");
Font font = new Font("Serif", Font.ITALIC, 36);
hourLabel.setFont(font);
minuteLabel.setFont(font);
secondLabel.setFont(font);
amPmLabel.setFont(font);
panel.add(hourLabel);
panel.add(new JLabel(":"));
panel.add(minuteLabel);
panel.add(new JLabel(":"));
panel.add(secondLabel);
panel.add(amPmLabel);
add(panel);
setVisible(true);
}
public void updateTime() {
Calendar calendar = Calendar.getInstance();
int hour = calendar.get(Calendar.HOUR);
int minute = calendar.get(Calendar.MINUTE);
int second = calendar.get(Calendar.SECOND);
int amPm = calendar.get(Calendar.AM_PM);
String amPmStr = amPm == Calendar.AM ? "AM" : "PM";
hourLabel.setText(String.format("%02d", hour));
minuteLabel.setText(String.format("%02d", minute));
secondLabel.setText(String.format("%02d", second));
amPmLabel.setText(amPmStr);
}
public static void main(String[] args) {
DigitalClock clock = new DigitalClock();
while (true) {
clock.updateTime();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// handle exception
}
}
}
}
```
This code creates a JFrame with four JLabels to display the time and AM/PM indicator. The updateTime() function uses the Calendar class to get the current time and updates the JLabels with the new time. Finally, the main method creates a DigitalClock object and continuously updates the time using the updateTime() function with a one second delay between each update.
相关推荐
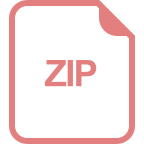
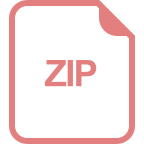
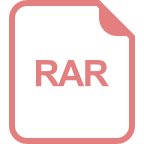















