const index = this.cart.findIndex((cartItem) => cartItem.name === item.name);改进这段代码
时间: 2023-06-13 18:02:18 浏览: 210
这段代码可以改进为使用 `Array.prototype.findIndex()` 的箭头函数简写形式,如下所示:
```
const index = this.cart.findIndex(cartItem => cartItem.name === item.name);
```
这样可以让代码更加简洁易读。另外,如果你使用了ES6的解构赋值,也可以将代码改写为:
```
const { name } = item;
const index = this.cart.findIndex(({ name: cartItemName }) => cartItemName === name);
```
这样可以更清晰地表达代码的意图,同时避免了多次访问 `item.name` 的问题。
相关问题
改进这段代码methods: { addToCart(item) { const index = this.cart.findIndex((cartItem) => cartItem.name == item.name); if (index == -1) { this.cart.push({ ...item, count: 1, }); } else { this.cart[index].count++; } }, removeFromCart(index) { this.cart.splice(index, 1); }, increase(index) { this.cart[index].count++; }, decrease(index) { if (this.cart[index].count > 1) { this.cart[index].count--; } }, },
这段代码中有一些问题。在 `addToCart` 方法中,如果商品已经存在于购物车中,它只是增加了商品的数量而没有更新购物车中该商品的其他属性。在 `increase` 方法中,语句 `this.cart[index].count ;` 没有对 `count` 属性进行任何操作。在 `decrease` 方法中,如果商品数量为 1,则不应删除该商品,而应该使其数量减少到 0。
下面是改进后的代码:
```
methods: {
addToCart(item) {
const index = this.cart.findIndex((cartItem) => cartItem.name == item.name);
if (index == -1) {
this.cart.push({
...item,
count: 1,
});
} else {
this.cart[index].count++;
}
},
removeFromCart(index) {
this.cart.splice(index, 1);
},
increase(index) {
this.cart[index].count++;
},
decrease(index) {
if (this.cart[index].count > 1) {
this.cart[index].count--;
} else {
this.removeFromCart(index);
}
},
},
```
在 `addToCart` 方法中,如果商品已经存在于购物车中,则只需增加其数量即可。在 `increase` 方法中,将商品数量加 1。在 `decrease` 方法中,如果商品数量大于 1,则将其数量减 1,否则删除该商品。
另外,代码中缺少对购物车中商品数量的总计的计算。可以添加一个计算属性来计算购物车中所有商品的数量总和。
```
computed: {
totalItems() {
return this.cart.reduce((total, item) => total + item.count, 0);
},
},
```
这个计算属性会返回购物车中所有商品的数量总和。可以将其用于显示购物车中所有商品的数量。
解释 methods: { async fn() { let { data } = await instance.get("/index.json"); this.arr = data.cart.products; // console.log(data.cart.products); }, startLogin() { this.$router.push({path: '/login'}) }, addToCart(product) { this.isCar = false; const cartItem = this.cartItems.find(item => item.product.id === product.id); if (cartItem) { cartItem.quantity++; } else { this.cartItems.push({ product, quantity: 1 }); } }, removeFromCart(item) { this.isCar = true; const index = this.cartItems.indexOf(item); this.cartItems.splice(index, 1); } }, computed: { totalPrice() { return this.cartItems.reduce((total, item) => total + item.product.price * item.quantity, 0); } }, components: {CarItem, Tabbar}, beforeMount() { this.fn(); }, };
这是一个 Vue.js 组件的代码,包括了一些方法和计算属性。
- `fn()` 是一个异步方法,使用 Axios 库发送 GET 请求,并请求获取 `/index.json` 的数据,将 `data.cart.products` 赋值给 `this.arr`,同时打印出 `data.cart.products`。
- `startLogin()` 是一个方法,在点击时将路由跳转到 `/login`。
- `addToCart(product)` 是一个方法,用于将商品添加到购物车中。如果购物车中已经存在该商品,则将该商品的数量加一,否则将该商品添加到 `cartItems` 数组中。
- `removeFromCart(item)` 是一个方法,用于从购物车中删除指定商品。首先将 `isCar` 设为 true,然后查找 `cartItems` 数组中与参数 `item` 对象相等的元素,删除该元素。
- `totalPrice()` 是一个计算属性,用于计算购物车中所有商品的总价。
在组件的生命周期中,使用 `beforeMount()` 钩子函数调用了 `fn()` 方法,获取商品列表数据。同时还引入了 `CarItem` 和 `Tabbar` 两个子组件。
阅读全文
相关推荐
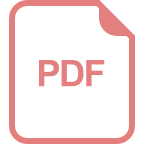
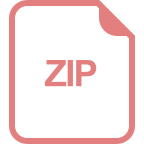
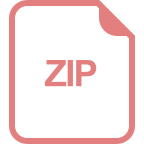













