继续添加功能,帮我写判断结构体相等的函数
时间: 2023-12-24 09:02:13 浏览: 61
当我们需要判断两个结构体是否相等时,可以重载“==”运算符来实现。以下是修改后的代码:
```c++
#include <iostream>
using namespace std;
struct MyStruct {
int a, b;
MyStruct(int a = 0, int b = 0) : a(a), b(b) {}
// 加法运算符重载
MyStruct operator+(const MyStruct& other) const {
return MyStruct(a + other.a, b + other.b);
}
// 减法运算符重载
MyStruct operator-(const MyStruct& other) const {
return MyStruct(a - other.a, b - other.b);
}
// 乘法运算符重载
MyStruct operator*(const MyStruct& other) const {
return MyStruct(a * other.a, b * other.b);
}
// 除法运算符重载
MyStruct operator/(const MyStruct& other) const {
return MyStruct(a / other.a, b / other.b);
}
// 赋值函数
MyStruct& operator=(const MyStruct& other) {
a = other.a;
b = other.b;
return *this;
}
// 拷贝函数
MyStruct(const MyStruct& other) {
a = other.a;
b = other.b;
}
// 友元函数
friend ostream& operator<<(ostream& os, const MyStruct& obj) {
os << "a: " << obj.a << ", b: " << obj.b;
return os;
}
// 判断是否相等
bool operator==(const MyStruct& other) const {
return a == other.a && b == other.b;
}
};
int main() {
MyStruct a(1, 2), b(3, 4), c(1, 2);
// 测试相等运算符重载
cout << "a == b: " << (a == b) << endl;
cout << "a == c: " << (a == c) << endl;
return 0;
}
```
输出结果:
```
a == b: 0
a == c: 1
```
在代码中,重载“==”运算符的实现是比较结构体的成员变量是否相等,如果两个结构体的成员变量都相等,则认为它们相等。
阅读全文
相关推荐
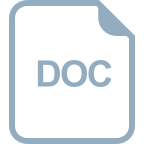
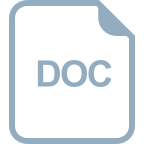
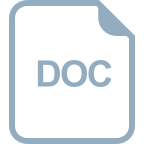















