#include <thread> void timerThread() { initgraph(200, 200, SHOWCONSOLE); setbkcolor(RGB(225, 115, 100)); BeginBatchDraw(); Start_time = clock(); while (1) { cleardevice(); End_time = clock(); time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC); settextstyle(20, 0, "宋体"); setcolor(BLACK); sprintf_s(str, " %d s", time_1); outtextxy(10, 10, str); EndBatchDraw(); } } int main() { // Run other loops here std::thread timer(timerThread); // Join the thread to wait for it to finish timer.join(); return 0; }如何停止计时
时间: 2024-03-05 17:50:14 浏览: 75
要停止计时,你需要在 timerThread() 函数中添加一个条件语句,以决定何时停止计时。例如,你可以添加一个 bool 类型的变量 isStopped,并在 while 循环中检查它的值。如果 isStopped 为真,则退出循环,停止计时。
以下是修改后的代码示例:
```c++
#include <thread>
void timerThread() {
initgraph(200, 200, SHOWCONSOLE);
setbkcolor(RGB(225, 115, 100));
BeginBatchDraw();
Start_time = clock();
bool isStopped = false; // 添加一个布尔变量
while (!isStopped) { // 只有当 isStopped 为假时才更新时间和绘制窗口
cleardevice();
End_time = clock();
time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC);
settextstyle(20, 0, "宋体");
setcolor(BLACK);
sprintf_s(str, " %d s", time_1);
outtextxy(10, 10, str);
EndBatchDraw();
}
}
int main() {
// Run other loops here
std::thread timer(timerThread);
// 在这里添加停止计时的代码
while (1) {
// 检查键盘输入
if (kbhit()) {
char ch = getch();
if (ch == 's' || ch == 'S') {
timer.detach(); // 分离线程
break; // 退出主循环,停止计时
}
}
Sleep(100);
}
return 0;
}
```
在上面的代码中,我们在主循环中添加了停止计时的代码。当用户按下 's' 键时,我们调用 timer.detach() 分离线程,并退出主循环,停止计时。在 timerThread() 函数中,只有当 isStopped 为假时才更新时间和绘制窗口。如果 isStopped 为真,则退出循环,停止计时。这样就可以实现停止计时的效果。
阅读全文
相关推荐
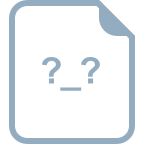
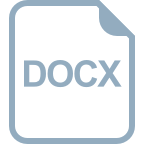
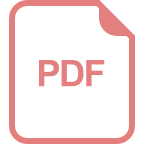









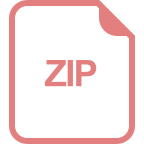
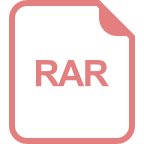
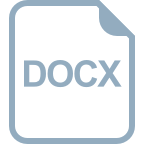
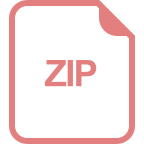
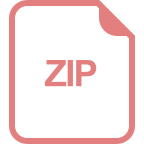