java8写一个将两个list按照多个key进行match的共通方法
时间: 2024-06-12 07:08:08 浏览: 13
可以使用Java8的Stream API和Lambda表达式来实现将两个List按照多个key进行match的共通方法。
以下是示例代码:
```java
import java.util.*;
import java.util.stream.Collectors;
public class ListMatcher {
public static <T> List<T> match(List<T> list1, List<T> list2, List<String> keys) {
return list1.stream()
.filter(item1 -> list2.stream().anyMatch(item2 -> keysMatch(item1, item2, keys)))
.collect(Collectors.toList());
}
private static <T> boolean keysMatch(T item1, T item2, List<String> keys) {
return keys.stream().allMatch(key -> Objects.equals(getPropertyValue(item1, key), getPropertyValue(item2, key)));
}
private static <T> Object getPropertyValue(T item, String key) {
try {
return item.getClass().getMethod(getGetterMethodName(key)).invoke(item);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
private static String getGetterMethodName(String propertyName) {
return "get" + propertyName.substring(0, 1).toUpperCase() + propertyName.substring(1);
}
}
```
这个方法接受两个List和一个key列表,返回匹配的结果List。
使用示例:
```java
public static void main(String[] args) {
List<Person> list1 = Arrays.asList(
new Person("Tom", "Smith", 20),
new Person("John", "Doe", 30),
new Person("Jane", "Doe", 25)
);
List<Person> list2 = Arrays.asList(
new Person("Tom", "Jones", 22),
new Person("John", "Doe", 30),
new Person("Jane", "Doe", 25)
);
List<String> keys = Arrays.asList("firstName", "lastName");
List<Person> result = ListMatcher.match(list1, list2, keys);
System.out.println(result);
}
```
其中Person类定义如下:
```java
public class Person {
private String firstName;
private String lastName;
private int age;
public Person(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public int getAge() {
return age;
}
@Override
public String toString() {
return "Person{" +
"firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
", age=" + age +
'}';
}
}
```
输出结果为:
```
[Person{firstName='John', lastName='Doe', age=30}, Person{firstName='Jane', lastName='Doe', age=25}]
```
这里使用了Person类作为示例,但实际上这个方法可以适用于任何具有公共getter方法的Java类。
相关推荐
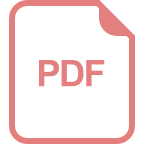
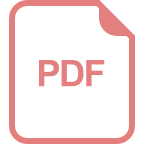
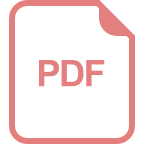













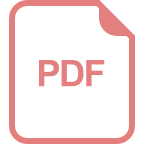
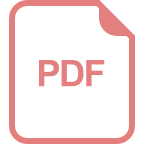