将tt100k_2021数据集中的annotations.json变成yolo的txt数据集
时间: 2023-06-26 15:06:03 浏览: 73
将tt100k_2021数据集中的annotations.json文件转换为YOLO格式的txt文件,可以按照以下步骤进行:
1. 首先,需要导入Python中的json和os模块,以及PIL库。
```python
import json
import os
from PIL import Image
```
2. 设置数据集路径和输出路径,以及类别标签。
```python
# 数据集路径
data_dir = "/path/to/tt100k_2021"
# 输出路径
output_dir = "/path/to/output"
# 类别标签
classes = ["car", "bus", "truck", "van", "others"]
```
3. 定义一个函数`convert_annotation`,用于将一张图片的标注信息转换为YOLO格式的标注信息。
```python
def convert_annotation(image_id, image_width, image_height, annotations):
txt_file = open(os.path.join(output_dir, f"{image_id}.txt"), "w")
for annotation in annotations:
if annotation["type"] in classes:
class_id = classes.index(annotation["type"])
bbox = annotation["bbox"]
x_center = bbox["x"] + bbox["w"] / 2
y_center = bbox["y"] + bbox["h"] / 2
width = bbox["w"]
height = bbox["h"]
x_center /= image_width
y_center /= image_height
width /= image_width
height /= image_height
txt_file.write(f"{class_id} {x_center} {y_center} {width} {height}\n")
txt_file.close()
```
该函数的输入参数包括图片ID、图片的宽度和高度,以及图片的标注信息。函数会将每个目标的类别转换为对应的类别ID,计算目标的中心点坐标、宽度和高度,并将其进行归一化处理后写入YOLO格式的txt文件中。
4. 遍历数据集中的所有图片,对每张图片调用`convert_annotation`函数进行转换。
```python
# 加载annotations.json文件
with open(os.path.join(data_dir, "annotations.json"), "r") as f:
annotations = json.load(f)
# 遍历所有图片
for image in annotations["imgs"].values():
image_id = image["id"]
image_path = os.path.join(data_dir, image["path"])
image_width, image_height = Image.open(image_path).size
convert_annotation(image_id, image_width, image_height, image["objects"])
```
5. 完成转换后,YOLO格式的txt文件将保存在输出路径中,每个文件的名称与对应的图片ID相同。
```python
# 输出转换完成提示信息
print(f"Convert annotations to YOLO format successfully! Output directory: {output_dir}")
```
完整代码示例:
```python
import json
import os
from PIL import Image
# 数据集路径
data_dir = "/path/to/tt100k_2021"
# 输出路径
output_dir = "/path/to/output"
# 类别标签
classes = ["car", "bus", "truck", "van", "others"]
def convert_annotation(image_id, image_width, image_height, annotations):
txt_file = open(os.path.join(output_dir, f"{image_id}.txt"), "w")
for annotation in annotations:
if annotation["type"] in classes:
class_id = classes.index(annotation["type"])
bbox = annotation["bbox"]
x_center = bbox["x"] + bbox["w"] / 2
y_center = bbox["y"] + bbox["h"] / 2
width = bbox["w"]
height = bbox["h"]
x_center /= image_width
y_center /= image_height
width /= image_width
height /= image_height
txt_file.write(f"{class_id} {x_center} {y_center} {width} {height}\n")
txt_file.close()
# 加载annotations.json文件
with open(os.path.join(data_dir, "annotations.json"), "r") as f:
annotations = json.load(f)
# 遍历所有图片
for image in annotations["imgs"].values():
image_id = image["id"]
image_path = os.path.join(data_dir, image["path"])
image_width, image_height = Image.open(image_path).size
convert_annotation(image_id, image_width, image_height, image["objects"])
# 输出转换完成提示信息
print(f"Convert annotations to YOLO format successfully! Output directory: {output_dir}")
```
相关推荐
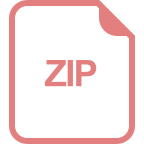
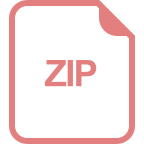














