使用ucos消息邮箱设计一个温度监测系统,编写两个任务task1()和task2()。其中,task1()负责定期采集温度数据(每间隔1分钟采集一次温度数据),并将采集到的温度数据进行保存;task2()负责对温度数据进行分析并输出温度值,当温度过高或过低时(当温度高于50度或温度低于10度时,发出报警信息),task2输出报警信息。task1和task2采用邮箱进行通信,当task1采集完温度数据后,往邮箱里面发一个信息告知task2任务温度数据采集已经完成,task2接收到task1发送的邮箱信息后,task2开始对温度数据进行分析、处理并输出。
时间: 2024-02-27 17:56:21 浏览: 60
下面是使用uC/OS-II消息邮箱实现的温度监测系统的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include "includes.h"
#define TEMP_HIGH 50
#define TEMP_LOW 10
OS_EVENT *Mbox; // 定义消息邮箱
void task1(void *pdata);
void task2(void *pdata);
int main(void)
{
OSInit();
Mbox = OSMboxCreate(NULL); // 创建消息邮箱
OSTaskCreate(task1, NULL, OSTaskStkSize, 10);
OSTaskCreate(task2, NULL, OSTaskStkSize, 11);
OSStart();
return 0;
}
void task1(void *pdata)
{
int temp = 0;
while (1)
{
// 模拟采集温度数据
temp = rand() % 100;
printf("Task1: Temperature data collected: %d\n", temp);
// 将采集到的温度数据发送到消息邮箱
OSMboxPost(Mbox, (void *)&temp);
OSTimeDlyHMSM(0, 1, 0, 0); // 每隔1分钟采集一次温度数据
}
}
void task2(void *pdata)
{
int temp = 0;
while (1)
{
// 等待消息邮箱中的温度数据
temp = *(int *)OSMboxPend(Mbox, 0, NULL);
printf("Task2: Temperature data received: %d\n", temp);
if (temp > TEMP_HIGH)
{
printf("Task2: Temperature is too high! Alarm!\n");
}
else if (temp < TEMP_LOW)
{
printf("Task2: Temperature is too low! Alarm!\n");
}
else
{
printf("Task2: Temperature is normal.\n");
}
}
}
```
在这个示例中,我们创建了两个任务:task1和task2。task1负责定期采集温度数据,并将采集到的温度数据发送到消息邮箱中;task2负责从消息邮箱中接收温度数据,并对温度数据进行处理和输出。当task2检测到温度过高或过低时,它会输出报警信息。
在程序中,我们使用了uC/OS-II提供的OSMboxCreate、OSMboxPost和OSMboxPend函数来创建消息邮箱、发送消息和接收消息。当task1采集完温度数据后,它使用OSMboxPost函数将温度数据发送到消息邮箱中;当task2等待消息邮箱中的数据时,它使用OSMboxPend函数从消息邮箱中接收温度数据。注意,在使用OSMboxPend函数时,我们将timeout参数设置为0,表示如果消息邮箱中没有数据,就立即返回。如果消息邮箱中有数据,OSMboxPend函数会返回指向数据的指针。
以上就是使用uC/OS-II消息邮箱实现温度监测系统的示例代码。当然,这只是一个简单的示例,实际应用中还需要考虑更多的因素,比如温度传感器的接口、数据处理算法等。
阅读全文
相关推荐
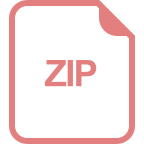
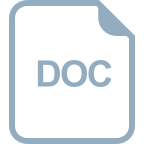
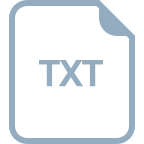
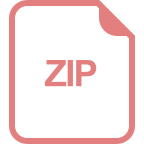
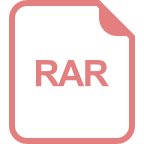
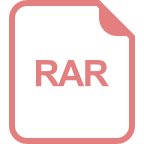
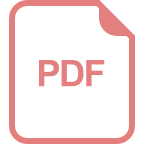
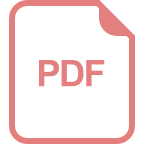
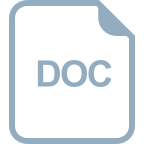
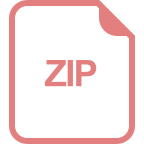
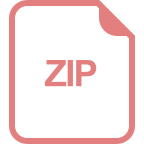
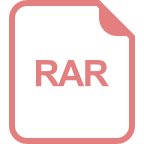
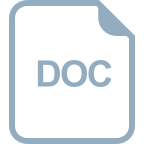


