apche hbase ResultScanner scanner 如何判断结果为空
时间: 2023-08-22 11:09:30 浏览: 130
当使用`ResultScanner`对象从HBase表中检索数据时,可以通过`next`方法从扫描器中获取下一个`Result`对象。如果表中没有更多的行可以扫描,则`next`方法将返回`null`。因此,可以通过检查`next`方法返回的结果是否为`null`来判断扫描结果是否为空。
以下是一个示例代码片段,可以演示如何使用`ResultScanner`对象并检查结果是否为空:
```
ResultScanner scanner = table.getScanner(scan);
Result result = scanner.next();
if (result == null) {
System.out.println("Scan result is empty.");
} else {
System.out.println("Scan result is not empty.");
}
```
在这个代码片段中,我们首先使用`getScanner`方法获取一个`ResultScanner`对象,然后使用`next`方法获取第一个`Result`对象。如果结果不为空,则输出“Scan result is not empty.”,否则输出“Scan result is empty.”。
相关问题
hbase 使用java写一个查询结果的多行数据保存在ResultScanner对象中,每行数据以Result对象 形式存储,Result中存储了多个Cell
HBase是一个基于列族的NoSQL分布式数据库,它非常适合处理大规模的数据。在HBase中,如果你想要从表中获取多行数据并将其保存到`ResultScanner`对象中,每个`Result`对象代表一行数据,并包含了该行的所有单元格(`Cells`),这些单元格通常包含键值对。
以下是使用Java操作HBase查询并保存多行数据的基本步骤:
```java
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.ScannerTimeoutException;
public void fetchDataFromHBase(String tableName) {
// 创建连接
Connection connection = ConnectionFactory.createConnection();
try {
// 定义Get请求,指定需要查询的行
Get get = new Get(Bytes.toBytes("rowKey1")); // "rowKey1"替换为你实际的行键
// 执行查询
ResultScanner scanner = table.getScanner(get);
// 存储结果
while (scanner.hasNext()) {
Result result = scanner.next();
List<Cell> cells = result.listCells(); // 获取当前行的所有Cell
for (Cell cell : cells) {
// 对每个cell进行操作,如获取其value等
byte[] value = cell.getValueArray();
// ...其他业务逻辑...
}
}
// 关闭扫描器
scanner.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (connection != null) {
try {
connection.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
使用hbase Java api完成以下操作: 1.建表userfllower 2.用户Mary有一个粉丝Alice,Alice关注列表中也存在Mary 3.用户Jack有两个粉丝Bob和Ben 4.Jack还关注了Mary 5.查看Jack被哪些人关注,Jack关注了谁 6.Bob取关了Jack 7.扫描全表数据 导入以下包完成import org.apache.hadoop.conf.Configuration;import org.apache.hadoop.hbase.;import org.apache.hadoop.hbase.client.;import org.apache.hadoop.hbase.util.Bytes;import java.io.IOException;
以下是使用 HBase Java API 完成以上操作的代码:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.*;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
import java.io.IOException;
public class HBaseExample {
private static final String TABLE_NAME = "userfollower";
private static final String COLUMN_FAMILY = "cf";
private static final String USER_QUALIFIER = "user";
private static final String FOLLOWER_QUALIFIER = "follower";
public static void main(String[] args) throws IOException {
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
Admin admin = connection.getAdmin();
// 1.建表userfollower
createTable(admin);
// 2.用户Mary有一个粉丝Alice,Alice关注列表中也存在Mary
addUserFollower(connection, "Mary", "Alice");
addUserFollower(connection, "Alice", "Mary");
// 3.用户Jack有两个粉丝Bob和Ben
addUserFollower(connection, "Jack", "Bob");
addUserFollower(connection, "Jack", "Ben");
// 4.Jack还关注了Mary
addUserFollower(connection, "Jack", "Mary");
// 5.查看Jack被哪些人关注,Jack关注了谁
scanUserFollowers(connection, "Jack");
// 6.Bob取关了Jack
removeUserFollower(connection, "Bob", "Jack");
// 7.扫描全表数据
scanTable(connection);
admin.close();
connection.close();
}
private static void createTable(Admin admin) throws IOException {
if (!admin.tableExists(TableName.valueOf(TABLE_NAME))) {
HTableDescriptor tableDescriptor = new HTableDescriptor(TableName.valueOf(TABLE_NAME));
HColumnDescriptor columnDescriptor = new HColumnDescriptor(COLUMN_FAMILY);
tableDescriptor.addFamily(columnDescriptor);
admin.createTable(tableDescriptor);
}
}
private static void addUserFollower(Connection connection, String user, String follower) throws IOException {
Table table = connection.getTable(TableName.valueOf(TABLE_NAME));
Put put = new Put(Bytes.toBytes(user));
put.addColumn(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(USER_QUALIFIER), Bytes.toBytes(user));
put.addColumn(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(FOLLOWER_QUALIFIER), Bytes.toBytes(follower));
table.put(put);
table.close();
}
private static void removeUserFollower(Connection connection, String user, String follower) throws IOException {
Table table = connection.getTable(TableName.valueOf(TABLE_NAME));
Delete delete = new Delete(Bytes.toBytes(user));
delete.addColumn(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(FOLLOWER_QUALIFIER), Bytes.toBytes(follower));
table.delete(delete);
table.close();
}
private static void scanUserFollowers(Connection connection, String user) throws IOException {
Table table = connection.getTable(TableName.valueOf(TABLE_NAME));
Scan scan = new Scan();
scan.addColumn(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(FOLLOWER_QUALIFIER));
scan.setRowPrefixFilter(Bytes.toBytes(user));
ResultScanner scanner = table.getScanner(scan);
System.out.println(user + " 的粉丝:");
for (Result result : scanner) {
byte[] followerBytes = result.getValue(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(FOLLOWER_QUALIFIER));
String follower = Bytes.toString(followerBytes);
System.out.println(follower);
}
scanner.close();
scan = new Scan();
scan.addColumn(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(USER_QUALIFIER));
scan.setRowPrefixFilter(Bytes.toBytes(user));
scanner = table.getScanner(scan);
System.out.println(user + " 关注的人:");
for (Result result : scanner) {
byte[] userBytes = result.getValue(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(USER_QUALIFIER));
String followedUser = Bytes.toString(userBytes);
System.out.println(followedUser);
}
scanner.close();
table.close();
}
private static void scanTable(Connection connection) throws IOException {
Table table = connection.getTable(TableName.valueOf(TABLE_NAME));
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
byte[] userBytes = result.getValue(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(USER_QUALIFIER));
String user = Bytes.toString(userBytes);
byte[] followerBytes = result.getValue(Bytes.toBytes(COLUMN_FAMILY), Bytes.toBytes(FOLLOWER_QUALIFIER));
String follower = Bytes.toString(followerBytes);
System.out.println(user + " 的粉丝:" + follower);
}
scanner.close();
table.close();
}
}
```
阅读全文
相关推荐
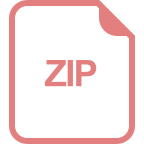
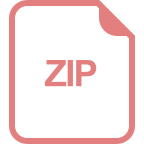
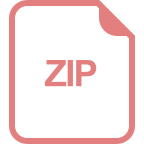
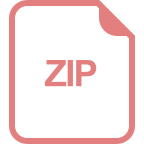
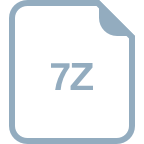
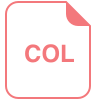
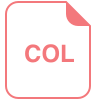
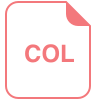
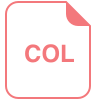







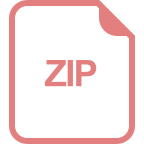
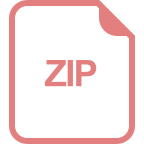