pinus client.ts 代码
时间: 2023-06-20 07:10:29 浏览: 142
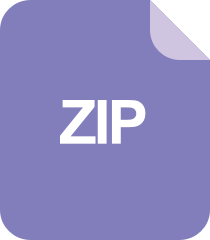
pinus-client
以下是一个基本的 Pinus 客户端代码示例,它使用 TypeScript 编写:
```typescript
import { createClient } from 'pomelo-protocol';
import { EventEmitter } from 'events';
class PinusClient extends EventEmitter {
private client: any;
private state: string;
constructor() {
super();
this.client = null;
this.state = 'closed';
}
public connect(host: string, port: number, cb?: Function) {
if (this.state !== 'closed') {
throw new Error('Invalid state: ' + this.state);
}
this.state = 'connecting';
const url = 'ws://' + host + ':' + port;
const client = createClient();
client.init({ host: host, port: port, log: true }, () => {
this.client = client;
this.state = 'connected';
this.emit('connect');
if (cb) {
cb();
}
});
client.on('close', () => {
this.state = 'closed';
this.client = null;
this.emit('disconnect');
});
client.on('io-error', (err: Error) => {
this.emit('error', err);
});
client.on('heartbeat timeout', () => {
this.emit('timeout');
});
}
public request(route: string, msg: any, cb?: Function) {
if (this.state !== 'connected') {
throw new Error('Invalid state: ' + this.state);
}
this.client.request(route, msg, (data: any) => {
if (cb) {
cb(data);
}
});
}
public notify(route: string, msg: any) {
if (this.state !== 'connected') {
throw new Error('Invalid state: ' + this.state);
}
this.client.notify(route, msg);
}
public disconnect() {
if (this.state !== 'connected') {
throw new Error('Invalid state: ' + this.state);
}
this.client.disconnect();
}
}
export default PinusClient;
```
在这个示例中,我们使用了 `pomelo-protocol` 库来创建 Pinus 客户端。`PinusClient` 类实现了以下方法:
- `connect(host: string, port: number, cb?: Function)`:连接到 Pinus 服务器。
- `request(route: string, msg: any, cb?: Function)`:向服务器发送请求。
- `notify(route: string, msg: any)`:向服务器发送通知。
- `disconnect()`:断开与服务器的连接。
在 `connect` 方法中,我们使用 `createClient` 函数来创建客户端,并在初始化完成后保存客户端对象。在客户端对象上监听 `close`、`io-error` 和 `heartbeat timeout` 事件,以便在客户端关闭、发生错误或超时时触发相应的事件。
在 `request` 和 `notify` 方法中,我们首先检查客户端状态是否为已连接,然后调用客户端对象的 `request` 或 `notify` 方法来向服务器发送请求或通知。
最后,在 `disconnect` 方法中,我们检查客户端状态是否为已连接,然后调用客户端对象的 `disconnect` 方法来断开与服务器的连接。
阅读全文
相关推荐
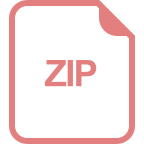
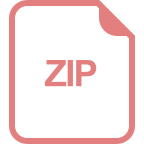














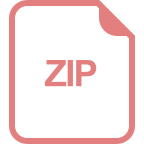