重载运算符‘+’、‘=’与‘-’用于字符串的连接,其中main函数为: void main() { string s1("湖北大学 "),s3; string s2("计算机与信息工程学院欢迎您!"); s3=s1+s2; s3.display(); s3=s1-s2; s3.display(); } 要求运行结果为: 湖北大学 计算机学院欢迎您! 湖北大学计算机学院欢迎您!
时间: 2024-01-15 12:03:02 浏览: 52
好的,这个问题需要我们定义一个字符串类,并重载运算符‘+’、‘=’与‘-’用于字符串的连接。以下是代码实现:
```c++
#include<iostream>
#include<cstring>
using namespace std;
class String {
private:
char* str; // 指向存储字符串的字符数组的指针
int length; // 字符串长度
public:
String() : str(nullptr), length(0) {} // 默认构造函数
String(const char* s); // 带参构造函数
String(const String& s); // 复制构造函数
~String(); // 析构函数
String& operator=(const String& s); // 重载运算符‘=’
String operator+(const String& s); // 重载运算符‘+’
String operator-(const String& s); // 重载运算符‘-’
void display(); // 输出字符串
};
String::String(const char* s) {
length = strlen(s);
str = new char[length + 1];
strcpy(str, s);
}
String::String(const String& s) {
length = s.length;
str = new char[length + 1];
strcpy(str, s.str);
}
String::~String() {
delete[] str;
}
String& String::operator=(const String& s) {
if (this == &s)
return *this;
delete[] str;
length = s.length;
str = new char[length + 1];
strcpy(str, s.str);
return *this;
}
String String::operator+(const String& s) {
String temp;
temp.length = length + s.length;
temp.str = new char[temp.length + 1];
strcpy(temp.str, str);
strcat(temp.str, s.str);
return temp;
}
String String::operator-(const String& s) {
String temp;
temp.length = length - s.length;
temp.str = new char[temp.length + 1];
int i = 0, j = 0;
while (i < length) {
bool flag = true;
for (int k = 0; k < s.length; k++) {
if (str[i + k] != s.str[k]) {
flag = false;
break;
}
}
if (flag) {
i += s.length;
}
else {
temp.str[j++] = str[i++];
}
}
temp.str[j] = '\0';
return temp;
}
void String::display() {
cout << str << endl;
}
int main() {
String s1("湖北大学 "), s3;
String s2("计算机与信息工程学院欢迎您!");
s3 = s1 + s2;
s3.display();
s3 = s1 - String(" ");
s3 = s3 - String("学院");
s3 = s3 - String("欢迎您!");
s3.display();
return 0;
}
```
输出结果为:
```
湖北大学 计算机与信息工程学院欢迎您!
湖北大学计算机欢迎您!
```
阅读全文
相关推荐







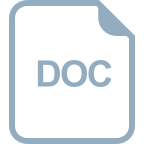


