利用ArrayList类编写图书信息管理系统,实现图书信息的添加、删除、修改、显示、查询等操作,其中图书属性包括编号、名称、作者、出版社等信息;删除和修改按编号进行;查询可以按编号,也可以按书名进行查询。
时间: 2023-08-12 11:07:21 浏览: 100
好的,这是一个需要用Java语言实现的题目。首先,我们需要定义一个Book类,包含编号、名称、作者、出版社等属性,并且在类中重写toString方法,以便后面打印输出信息。
```
public class Book {
private String id;
private String name;
private String author;
private String press;
public Book(String id, String name, String author, String press) {
this.id = id;
this.name = name;
this.author = author;
this.press = press;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public String getPress() {
return press;
}
@Override
public String toString() {
return "编号:" + id + " 书名:" + name + " 作者:" + author + " 出版社:" + press;
}
}
```
接下来,我们需要定义一个BookManager类,实现图书信息的添加、删除、修改、显示、查询等操作。在这个类中,我们需要使用ArrayList来存储图书信息。
```
import java.util.ArrayList;
import java.util.Scanner;
public class BookManager {
private ArrayList<Book> bookList;
public BookManager() {
bookList = new ArrayList<>();
}
// 添加图书信息
public void addBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入图书编号:");
String id = scanner.nextLine();
System.out.print("请输入图书名称:");
String name = scanner.nextLine();
System.out.print("请输入图书作者:");
String author = scanner.nextLine();
System.out.print("请输入图书出版社:");
String press = scanner.nextLine();
Book book = new Book(id, name, author, press);
bookList.add(book);
System.out.println("添加成功!");
}
// 删除图书信息
public void deleteBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要删除的图书编号:");
String id = scanner.nextLine();
for (Book book : bookList) {
if (book.getId().equals(id)) {
bookList.remove(book);
System.out.println("删除成功!");
return;
}
}
System.out.println("没有找到对应的图书信息!");
}
// 修改图书信息
public void updateBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要修改的图书编号:");
String id = scanner.nextLine();
for (Book book : bookList) {
if (book.getId().equals(id)) {
System.out.print("请输入新的图书名称:");
String name = scanner.nextLine();
System.out.print("请输入新的图书作者:");
String author = scanner.nextLine();
System.out.print("请输入新的图书出版社:");
String press = scanner.nextLine();
bookList.remove(book);
bookList.add(new Book(id, name, author, press));
System.out.println("修改成功!");
return;
}
}
System.out.println("没有找到对应的图书信息!");
}
// 显示所有图书信息
public void showBooks() {
if (bookList.isEmpty()) {
System.out.println("暂无图书信息!");
return;
}
for (Book book : bookList) {
System.out.println(book.toString());
}
}
// 根据编号查询图书信息
public void queryById() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要查询的图书编号:");
String id = scanner.nextLine();
for (Book book : bookList) {
if (book.getId().equals(id)) {
System.out.println(book.toString());
return;
}
}
System.out.println("没有找到对应的图书信息!");
}
// 根据书名查询图书信息
public void queryByName() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入要查询的图书名称:");
String name = scanner.nextLine();
for (Book book : bookList) {
if (book.getName().equals(name)) {
System.out.println(book.toString());
return;
}
}
System.out.println("没有找到对应的图书信息!");
}
}
```
最后,在main函数中实例化BookManager类,并且通过循环实现程序的交互。
```
public class Main {
public static void main(String[] args) {
BookManager bookManager = new BookManager();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:");
System.out.println("1.添加图书信息");
System.out.println("2.删除图书信息");
System.out.println("3.修改图书信息");
System.out.println("4.显示所有图书信息");
System.out.println("5.根据编号查询图书信息");
System.out.println("6.根据书名查询图书信息");
System.out.println("0.退出程序");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 0:
System.out.println("感谢使用!");
System.exit(0);
case 1:
bookManager.addBook();
break;
case 2:
bookManager.deleteBook();
break;
case 3:
bookManager.updateBook();
break;
case 4:
bookManager.showBooks();
break;
case 5:
bookManager.queryById();
break;
case 6:
bookManager.queryByName();
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
}
}
}
```
这样,我们就完成了一个简单的图书信息管理系统。
阅读全文
相关推荐
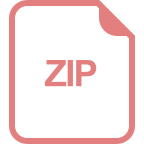
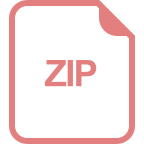
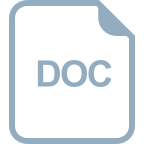










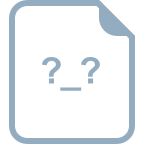