ECC加解密算法使用c语言,写一个复杂完整的
时间: 2024-12-23 20:22:10 浏览: 14
由于在C语言中直接编写ECC加解密的全部细节会相当繁琐,我将提供一个基本框架,展示如何使用OpenSSL库,这是一个广泛用于C语言的加密库。请注意,真正的生产环境中,你还需要考虑更多边缘情况和错误处理。
```c
#include <openssl/bio.h>
#include <openssl/err.h>
#include <openssl/ec.h>
#include <openssl/pem.h>
// 生成EC密钥对
EC_KEY* generate_keys(void) {
EC_KEY *ecdh = EC_KEY_new_by_curve_name(NID_secp256r1);
if (!ecdh) {
perror("Failed to create ECDH key");
return NULL;
}
BIGNUM *pub_num, *priv_num;
priv_num = BN_new();
pub_num = BN_new();
if (!BN_generate_prime(priv_num, 256, NULL)) {
EC_KEY_free(ecdh);
return NULL;
}
EC_POINT *pub_point;
if ((pub_point = EC_POINT_new(ecdh)) == NULL) {
BN_clear_free(priv_num);
EC_KEY_free(ecdh);
return NULL;
}
if (!EC_POINT_mul(ecdh, pub_point, priv_num, NULL, NULL, NULL)) {
BN_clear_free(pub_num);
BN_clear_free(priv_num);
EC_POINT_free(pub_point);
EC_KEY_free(ecdh);
return NULL;
}
EC_KEY_set_public_key(ecdh, pub_point);
// 私钥存储
ECDSA *ecdsa = ECDSA_new();
if (!ecdsa || !ECDSA_set_private_key(ecdh, priv_num)) {
EC_KEY_free(ecdh);
ECDSA_free(ecdsa);
return NULL;
}
EC_KEY_free(ecdh);
return ecdsa;
}
// 加密函数
char* encrypt(const char* plaintext, EC_KEY* ecdsa) {
unsigned char ciphertext[EVP_MAX_MD_SIZE];
int len;
BIO* bio_out = BIO_new(BIO_s_mem());
if (!bio_out) {
perror("Failed to create BIO for output");
return NULL;
}
if (!ECDSA_sign(0, plaintext, strlen(plaintext), bio_out, NULL, ecdsa)) {
BIO_free_all(bio_out);
return NULL;
}
len = BIO_get_mem_data(bio_out, &ciphertext);
BIO_free_all(bio_out);
return (char*) ciphertext;
}
// 解密函数
int decrypt(char* ciphertext, size_t ciphertext_len, EC_KEY* ecdsa) {
unsigned char signature[EVP_MAX_MD_SIZE];
int sig_len;
BIO* bio_in = BIO_new_mem_buf((void*)ciphertext, ciphertext_len);
if (!bio_in) {
perror("Failed to create BIO for input");
return -1;
}
if (!ECDSA_verify(0, ciphertext, ciphertext_len, bio_in, ecdsa)) {
BIO_free_all(bio_in);
return -1;
}
sig_len = BIO_read(bio_in, signature, sizeof(signature));
BIO_free_all(bio_in);
return sig_len; // 返回解密后的长度(通常就是原始数据长度)
}
int main(int argc, char** argv) {
EC_KEY* ecdsa = generate_keys();
if (!ecdsa) {
perror("Failed to generate keys");
return 1;
}
char* plaintext = "Hello, ECC!";
char* ciphertext = encrypt(plaintext, ecdsa);
if (!ciphertext) {
perror("Failed to encrypt");
EC_KEY_free(ecdsa);
return 1;
}
// 解密并打印结果
int decrypted_len = decrypt(ciphertext, strlen(ciphertext), ecdsa);
if (decrypted_len > 0) {
printf("Decrypted message: %.*s\n", (int)decrypted_len, ciphertext);
} else {
printf("Decryption failed.\n");
}
EC_KEY_free(ecdsa);
return 0;
}
```
这个代码示例展示了创建ECC密钥、加密、解密的基本流程,但实际应用时你需要完善错误处理和内存管理。另外,注意ECDH部分仅用于交换信息,而ECDSA用于数字签名,如果你需要的是保密通信,应该使用ECDH。
阅读全文
相关推荐



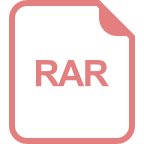













