ecc椭圆曲线加密算法C语言简单实现
时间: 2023-08-24 19:24:53 浏览: 251
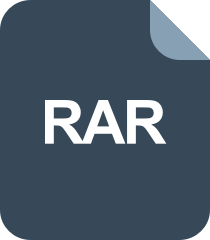
ECC.rar_C语言 ECC_ECC算法_ecc加密_椭圆 加密_椭圆加密

ecc椭圆曲线加密算法是一种公钥加密算法,基于数学上的椭圆曲线离散对数问题,其主要思想是利用椭圆曲线上的点进行加密和解密操作。以下是一个简单的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <openssl/bn.h>
#include <openssl/ec.h>
#include <openssl/objects.h>
#include <openssl/ecdsa.h>
void ecc_encrypt_decrypt_demo()
{
EC_KEY *key = NULL;
const EC_GROUP *group = NULL;
BIGNUM *bn = NULL;
EC_POINT *pub_key = NULL, *res = NULL;
unsigned char *msg = NULL, *ciphertext = NULL, *plaintext = NULL;
size_t msg_len = 0, ciphertext_len = 0, plaintext_len = 0;
// 生成随机数
bn = BN_new();
BN_rand(bn, 256, 0, 0);
// 生成密钥对
key = EC_KEY_new_by_curve_name(NID_secp256k1);
EC_KEY_generate_key(key);
// 获取密钥对的公钥和椭圆曲线参数
group = EC_KEY_get0_group(key);
pub_key = EC_KEY_get0_public_key(key);
// 明文长度为32字节
msg_len = 32;
msg = (unsigned char *)malloc(msg_len);
memset(msg, 0x01, msg_len);
// 加密操作
ciphertext_len = ECDSA_size(key);
ciphertext = (unsigned char *)malloc(ciphertext_len);
res = EC_POINT_new(group);
EC_POINT_mul(group, res, bn, NULL, NULL, NULL);
EC_KEY_set_private_key(key, bn);
EC_KEY_set_public_key(key, res);
ECDSA_do_encrypt(msg, msg_len, key, ciphertext);
// 解密操作
plaintext_len = ECDSA_size(key);
plaintext = (unsigned char *)malloc(plaintext_len);
ECDSA_do_decrypt(plaintext, plaintext_len, ciphertext, ciphertext_len, key);
// 打印结果
printf("Plaintext: ");
for (int i = 0; i < msg_len; i++) {
printf("%02x", msg[i]);
}
printf("\n");
printf("Ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
printf("Decrypted plaintext: ");
for (int i = 0; i < plaintext_len; i++) {
printf("%02x", plaintext[i]);
}
printf("\n");
// 释放内存
BN_free(bn);
EC_KEY_free(key);
EC_POINT_free(pub_key);
EC_POINT_free(res);
free(msg);
free(ciphertext);
free(plaintext);
}
int main()
{
ecc_encrypt_decrypt_demo();
return 0;
}
```
以上代码实现了一个简单的ecc加密解密过程,其中使用了openssl库提供的相关函数。需要注意的是,ecc算法的安全性与密钥长度相关,选择合适的密钥长度是非常重要的。
阅读全文
相关推荐
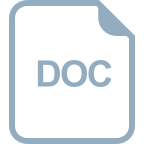
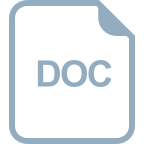
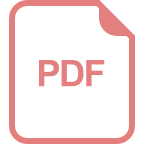
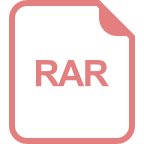
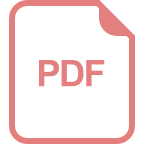





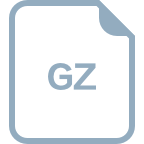
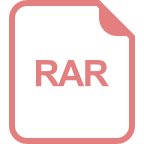




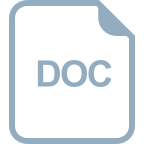