c++的vector<int>使用length函数计算元素个数有什么问题?给出例子解释length函数的使用
时间: 2023-05-31 07:03:51 浏览: 62
在C++中,vector容器使用的是size()函数来计算元素个数,而不是length()函数。使用length()函数会导致编译错误。
例如:
```c++
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> arr{1, 2, 3, 4, 5};
cout << arr.length() << endl; //编译错误,vector没有length()函数
return 0;
}
```
输出:
```
error: no member named 'length' in 'std::__1::vector<int, std::__1::allocator<int> >'
```
正确的做法是使用size()函数:
```c++
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> arr{1, 2, 3, 4, 5};
cout << arr.size() << endl; //输出5
return 0;
}
```
输出:
```
5
```
相关问题
no member named 'length' in 'std::vector<int, std::allocator<int>>'
这个错误是因为在C++中,`std::vector`没有名为`length`的成员函数。正确的成员函数是`size()`。所以,你需要将`nums.length()`改为`nums.size()`。
以下是一个示例代码,演示了如何使用`std::vector`的`size()`函数来获取向量的长度:
```cpp
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {4, 5, 1, 4, 0};
int length = nums.size();
std::cout << "Length of the vector: " << length << std::endl;
return 0;
}
```
这段代码将输出:Length of the vector: 5
#include <iostream>#include <vector>#include <algorithm>using namespace std;int countGoodRectangles(vector<vector<int>>& rectangles) { int maxLen = 0, count = 0; for (vector<int>& rectangle : rectangles) { int len = min(rectangle[0], rectangle[1]); if (len > maxLen) { maxLen = len; count = 1; } else if (len == maxLen) { count++; } } return count;}int main() { vector<vector<int>> rectangles = {{5,8},{3,9},{5,12},{16,5}}; int count = countGoodRectangles(rectangles); cout << "Number of rectangles that can be cut into squares with maximum length: " << count << endl; return 0;}该代码如何改成自己输入二维数组内容
你可以通过以下方式修改代码,使其可以接受用户输入的二维数组内容:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int countGoodRectangles(vector<vector<int>>& rectangles) {
int maxLen = 0, count = 0;
for (vector<int>& rectangle : rectangles) {
int len = min(rectangle[0], rectangle[1]);
if (len > maxLen) {
maxLen = len;
count = 1;
} else if (len == maxLen) {
count++;
}
}
return count;
}
int main() {
int rows, cols;
cout << "Enter number of rows: ";
cin >> rows;
cout << "Enter number of columns: ";
cin >> cols;
vector<vector<int>> rectangles(rows, vector<int>(cols));
cout << "Enter elements of the array:" << endl;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cin >> rectangles[i][j];
}
}
int count = countGoodRectangles(rectangles);
cout << "Number of rectangles that can be cut into squares with maximum length: " << count << endl;
return 0;
}
```
这段代码通过 `cin` 函数接受用户输入的行数和列数,然后创建一个大小为 `rows x cols` 的二维向量 `rectangles`。接下来,程序提示用户输入二维数组的元素,并依次读入。最后,程序调用 `countGoodRectangles` 函数计算结果,然后输出。
相关推荐
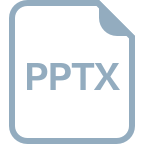
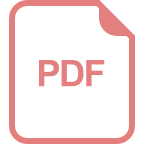
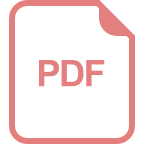












