使用freemarker、Itext进行Html转Pdf
时间: 2023-12-26 09:03:46 浏览: 237
使用Freemarker和iText可以方便地将HTML转换为PDF。下面是一个基本的示例:
1. 首先,您需要引入iText和freemarker的依赖项。如果您使用的是Maven,可以添加以下依赖项到pom.xml文件中:
```
<dependency>
<groupId>com.lowagie</groupId>
<artifactId>itext</artifactId>
<version>2.1.7</version>
</dependency>
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.31</version>
</dependency>
```
2. 创建Freemarker模板,例如:
```
<html>
<head>
<title>${title}</title>
</head>
<body>
<h1>${heading}</h1>
<p>${content}</p>
</body>
</html>
```
3. 创建Java类来生成PDF文件。例如:
```
import java.io.*;
import java.util.*;
import com.lowagie.text.*;
import com.lowagie.text.pdf.*;
import freemarker.template.*;
public class HtmlToPdf {
public static void main(String[] args) throws Exception {
// 创建数据模型
Map<String, Object> data = new HashMap<String, Object>();
data.put("title", "My PDF Document");
data.put("heading", "Hello World");
data.put("content", "This is my first PDF document.");
// 创建Freemarker模板
Configuration cfg = new Configuration(Configuration.VERSION_2_3_31);
cfg.setClassForTemplateLoading(HtmlToPdf.class, "/");
Template template = cfg.getTemplate("template.ftl");
// 将Freemarker模板渲染为HTML
StringWriter writer = new StringWriter();
template.process(data, writer);
String html = writer.toString();
// 创建PDF文档
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream("output.pdf"));
document.open();
// 将HTML转换为PDF
InputStream is = new ByteArrayInputStream(html.getBytes());
XMLWorkerHelper.getInstance().parseXHtml(writer, document, is);
// 关闭PDF文档
document.close();
}
}
```
这个示例将生成一个名为“output.pdf”的PDF文件,其中包含一个标题为“My PDF Document”的页面,以及一个标题为“Hello World”和内容为“This is my first PDF document.”的段落。
请注意,此示例仅是一个基本示例。您可以根据需要修改模板和Java代码以生成更复杂的PDF文件。
阅读全文
相关推荐
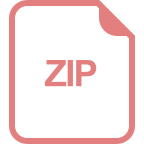
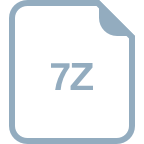
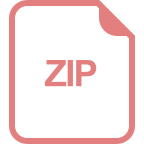
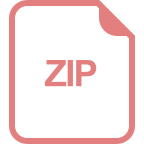
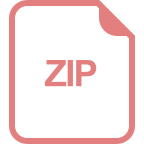
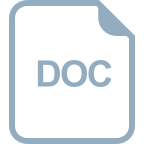


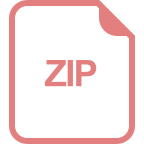
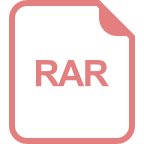
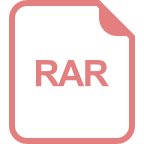
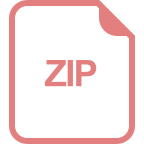
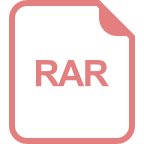
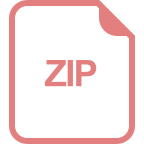
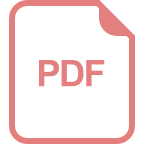
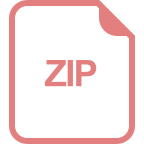