c语言完成无向图的创建和DFS(深度优先遍历)和BFS(广度优先遍历)
时间: 2023-06-13 17:03:18 浏览: 198
以下是使用邻接矩阵表示无向图,以及实现DFS和BFS遍历的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_VERTEX_NUM 100 // 最大顶点数
// 邻接矩阵结构体
typedef struct {
int vertex[MAX_VERTEX_NUM]; // 顶点数组
int edge[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 边的矩阵
int vertex_num; // 顶点个数
int edge_num; // 边的个数
} Graph;
// 初始化图
void init_graph(Graph *graph) {
graph->vertex_num = 0;
graph->edge_num = 0;
for (int i = 0; i < MAX_VERTEX_NUM; i++) {
graph->vertex[i] = 0;
for (int j = 0; j < MAX_VERTEX_NUM; j++) {
graph->edge[i][j] = 0;
}
}
}
// 添加顶点
void add_vertex(Graph *graph, int v) {
graph->vertex[graph->vertex_num++] = v;
}
// 添加边
void add_edge(Graph *graph, int v1, int v2) {
graph->edge[v1][v2] = 1;
graph->edge[v2][v1] = 1;
graph->edge_num++;
}
// DFS遍历
void dfs(Graph *graph, int v, bool *visited) {
visited[v] = true;
printf("%d ", graph->vertex[v]);
for (int i = 0; i < graph->vertex_num; i++) {
if (graph->edge[v][i] == 1 && !visited[i]) {
dfs(graph, i, visited);
}
}
}
// BFS遍历
void bfs(Graph *graph, int v, bool *visited) {
int queue[MAX_VERTEX_NUM];
int front = 0, rear = 0;
visited[v] = true;
printf("%d ", graph->vertex[v]);
queue[rear++] = v;
while (front < rear) {
int cur = queue[front++];
for (int i = 0; i < graph->vertex_num; i++) {
if (graph->edge[cur][i] == 1 && !visited[i]) {
visited[i] = true;
printf("%d ", graph->vertex[i]);
queue[rear++] = i;
}
}
}
}
int main() {
Graph graph;
init_graph(&graph);
add_vertex(&graph, 0);
add_vertex(&graph, 1);
add_vertex(&graph, 2);
add_vertex(&graph, 3);
add_vertex(&graph, 4);
add_edge(&graph, 0, 1);
add_edge(&graph, 0, 2);
add_edge(&graph, 1, 3);
add_edge(&graph, 2, 4);
bool visited[MAX_VERTEX_NUM] = {0};
printf("DFS遍历:");
dfs(&graph, 0, visited);
printf("\nBFS遍历:");
for (int i = 0; i < MAX_VERTEX_NUM; i++) {
visited[i] = false;
}
bfs(&graph, 0, visited);
printf("\n");
return 0;
}
```
在上面的代码中,我们使用邻接矩阵来表示无向图,其中`vertex`数组存储顶点,`edge`矩阵存储边。`add_vertex`函数用于添加顶点,`add_edge`函数用于添加边。`dfs`函数实现DFS遍历,`bfs`函数实现BFS遍历,`visited`数组用于标记已访问的顶点。在主函数中,我们创建了一个无向图,并进行了DFS和BFS遍历。
阅读全文
相关推荐
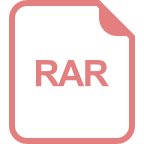
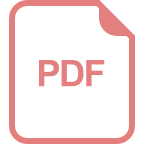















