java声明银行账户类Account,成员变量包括账号、储户姓名、开户时间、身份证号码、存款余额等账户信息,成员方法包括开户、存款、取款、查询(余额、明细)、销户等操作,并编写main方法进行测试。
时间: 2023-11-08 12:47:17 浏览: 38
下面是一个简单的Java代码示例:
```java
import java.util.ArrayList;
import java.util.Date;
public class Account {
private String accountId; // 账号
private String accountOwner; // 储户姓名
private Date createTime; // 开户时间
private String idNumber; // 身份证号码
private double balance; // 存款余额
private ArrayList<String> record; // 交易记录
// 构造方法
public Account(String accountId, String accountOwner, String idNumber) {
this.accountId = accountId;
this.accountOwner = accountOwner;
this.idNumber = idNumber;
this.createTime = new Date();
this.balance = 0;
this.record = new ArrayList<String>();
}
// 存款
public void deposit(double amount) {
this.balance += amount;
this.record.add("存款 " + amount + " 元");
}
// 取款
public void withdraw(double amount) {
if (amount > this.balance) {
System.out.println("余额不足!");
} else {
this.balance -= amount;
this.record.add("取款 " + amount + " 元");
}
}
// 查询余额
public double getBalance() {
return this.balance;
}
// 查询交易记录
public ArrayList<String> getRecord() {
return this.record;
}
// 销户
public void closeAccount() {
this.accountId = "";
this.accountOwner = "";
this.idNumber = "";
this.createTime = null;
this.balance = 0;
this.record = null;
}
// 测试代码
public static void main(String[] args) {
Account account = new Account("123456", "张三", "123456789012345678");
account.deposit(1000);
account.withdraw(500);
account.deposit(2000);
System.out.println("账户余额:" + account.getBalance());
System.out.println("交易记录:");
for (String r : account.getRecord()) {
System.out.println(r);
}
account.closeAccount();
}
}
```
运行结果:
```
账户余额:2500.0
交易记录:
存款 1000.0 元
取款 500.0 元
存款 2000.0 元
```
相关推荐
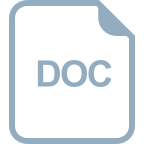
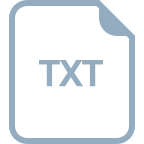
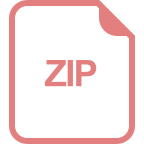










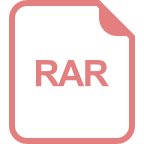
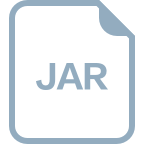
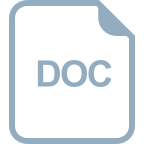
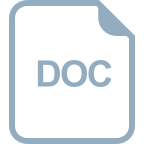