定义接口或类 Shape,定义求周长的方法length()。 定义如下类,实现接口Shape或父类Shape的方法。 (1)三角形类Triangle (2)长方形类Rectangle (3)圆形类Circle等。 定义测试类ShapeTest,用Shape接口(或类)定义变量shape,用其指向不同类形的对象,输出各种图形的周长。并为其他的Shape接口实现类提供良好的扩展性。 提示: 计算圆周长时PI取3.14。
时间: 2023-07-10 18:11:57 浏览: 73
好的,这是一个Python的面向对象编程的问题,我可以帮你解答。
首先,我们需要定义Shape接口或类,包括一个求周长的方法length(),代码如下:
```python
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def length(self):
pass
```
接着,我们需要定义不同的形状类,分别实现Shape接口或继承Shape类,并实现length()方法,代码如下:
```python
class Triangle(Shape):
def __init__(self, a, b, c):
self.a = a
self.b = b
self.c = c
def length(self):
return self.a + self.b + self.c
class Rectangle(Shape):
def __init__(self, a, b):
self.a = a
self.b = b
def length(self):
return 2 * (self.a + self.b)
class Circle(Shape):
def __init__(self, r):
self.r = r
def length(self):
return 2 * 3.14 * self.r
```
最后,我们可以定义ShapeTest测试类,用Shape接口定义变量shape,用其指向不同类形的对象,输出各种图形的周长,代码如下:
```python
if __name__ == '__main__':
shape1 = Triangle(3, 4, 5)
shape2 = Rectangle(2, 4)
shape3 = Circle(5)
print("Triangle length: ", shape1.length())
print("Rectangle length: ", shape2.length())
print("Circle length: ", shape3.length())
```
这样,我们就完成了该问题的解答。值得注意的是,这里使用了抽象基类(ABC)来定义Shape接口,这是Python中的一种常见方法。同时,我们也实现了良好的扩展性,如果有其他的形状类需要实现Shape接口,只需要继承Shape类并实现length()方法即可。
相关推荐
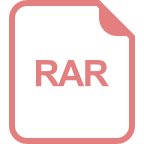
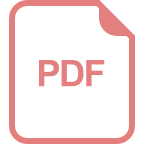












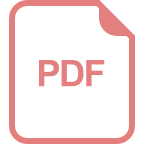
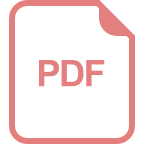