用c++实现基于openssl的对称加密与解密
时间: 2023-12-17 09:02:33 浏览: 138
以下是一个简单的示例代码,实现了基于 OpenSSL 的对称加密和解密:
```c++
#include <iostream>
#include <openssl/evp.h>
#include <openssl/aes.h>
#include <openssl/rand.h>
#define KEY_SIZE 32
#define IV_SIZE 16
int aes_encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext) {
EVP_CIPHER_CTX *ctx;
int len, ciphertext_len;
if (!(ctx = EVP_CIPHER_CTX_new())) return -1;
if (1 != EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), nullptr, key, iv))
return -1;
if (1 != EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len))
return -1;
ciphertext_len = len;
if (1 != EVP_EncryptFinal_ex(ctx, ciphertext + len, &len)) return -1;
ciphertext_len += len;
EVP_CIPHER_CTX_free(ctx);
return ciphertext_len;
}
int aes_decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
EVP_CIPHER_CTX *ctx;
int len, plaintext_len, ret;
if (!(ctx = EVP_CIPHER_CTX_new())) return -1;
if (1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), nullptr, key, iv))
return -1;
if (1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len))
return -1;
plaintext_len = len;
ret = EVP_DecryptFinal_ex(ctx, plaintext + len, &len);
EVP_CIPHER_CTX_free(ctx);
if (ret > 0) {
plaintext_len += len;
return plaintext_len;
} else {
return -1;
}
}
int main() {
unsigned char key[KEY_SIZE], iv[IV_SIZE];
unsigned char plaintext[] = "Hello, world!";
unsigned char ciphertext[sizeof(plaintext) + AES_BLOCK_SIZE];
unsigned char decryptedtext[sizeof(plaintext)];
int decryptedtext_len, ciphertext_len;
// Generate random key and iv
RAND_bytes(key, KEY_SIZE);
RAND_bytes(iv, IV_SIZE);
// Encrypt the plaintext
ciphertext_len = aes_encrypt(plaintext, sizeof(plaintext), key, iv, ciphertext);
// Decrypt the ciphertext
decryptedtext_len = aes_decrypt(ciphertext, ciphertext_len, key, iv, decryptedtext);
// Print the results
std::cout << "Plaintext: " << plaintext << std::endl;
std::cout << "Ciphertext: " << ciphertext << std::endl;
std::cout << "Decrypted text: " << decryptedtext << std::endl;
return 0;
}
```
该代码使用了 AES-256-CBC 对称加密算法,生成一个随机的 256 位密钥和 128 位 IV。加密和解密时使用相同的密钥和 IV。注意,此代码中的明文和密文都不包括终止符。
阅读全文
相关推荐


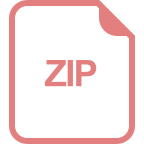
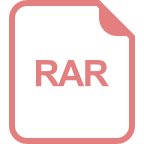
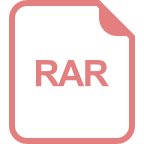
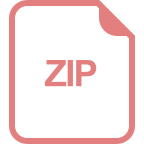
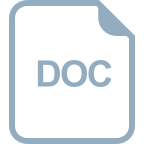
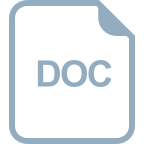
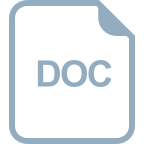
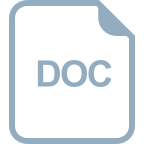





