用c++使用链表实现下面各种排序算法,写出代码内并进行比较。 排序算法: 1、插入排序 2、冒泡排序 3、快速排序 4、简单选择排序 5、其他 要求: 1、测试数据分成三类:正序、逆序、随机数据 2、对于这三类数据,比较上述排序算法中关键字的比较次数和移动次数(其 中关键字交换计为 3 次移动)。 3、对于这三类数据,比较上述排序算法中不同算法的执行时间,精确到微秒 (选作) 4、对 2 和 3 的结果进行分析,验证上述各种算法的时间复杂度 编写测试 main()函数测试排序算法的正确性
时间: 2023-08-31 18:33:15 浏览: 95
好的,以下是使用链表实现各种排序算法的代码,并且进行了比较:
```c++
#include <iostream>
#include <ctime>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 插入排序
ListNode* insertionSortList(ListNode* head) {
if (!head || !head->next) return head;
ListNode* dummy = new ListNode(-1);
dummy->next = head;
ListNode* cur = head->next;
head->next = NULL;
while (cur) {
ListNode* p = dummy;
while (p->next && p->next->val < cur->val) {
p = p->next;
}
ListNode* next = cur->next;
cur->next = p->next;
p->next = cur;
cur = next;
}
ListNode* newHead = dummy->next;
delete dummy;
return newHead;
}
// 冒泡排序
ListNode* bubbleSortList(ListNode* head) {
if (!head || !head->next) return head;
ListNode* dummy = new ListNode(-1);
dummy->next = head;
bool swapped = true;
while (swapped) {
swapped = false;
ListNode* p = dummy;
while (p->next && p->next->next) {
if (p->next->val > p->next->next->val) {
ListNode* q = p->next->next;
p->next->next = q->next;
q->next = p->next;
p->next = q;
swapped = true;
}
p = p->next;
}
}
ListNode* newHead = dummy->next;
delete dummy;
return newHead;
}
// 快速排序
ListNode* quickSortList(ListNode* head) {
if (!head || !head->next) return head;
ListNode* pivot = head;
ListNode* p = head->next;
ListNode* less = new ListNode(-1);
ListNode* greater = new ListNode(-1);
ListNode* pLess = less;
ListNode* pGreater = greater;
while (p) {
if (p->val < pivot->val) {
pLess->next = p;
pLess = pLess->next;
}
else {
pGreater->next = p;
pGreater = pGreater->next;
}
p = p->next;
}
pLess->next = NULL;
pGreater->next = NULL;
less = quickSortList(less->next);
greater = quickSortList(greater->next);
pivot->next = greater;
if (less) {
pLess = less;
while (pLess->next) {
pLess = pLess->next;
}
pLess->next = pivot;
return less;
}
else {
return pivot;
}
}
// 简单选择排序
ListNode* selectionSortList(ListNode* head) {
if (!head || !head->next) return head;
ListNode* dummy = new ListNode(-1);
dummy->next = head;
ListNode* p = head;
while (p->next) {
ListNode* minNode = p;
ListNode* q = p->next;
while (q) {
if (q->val < minNode->val) {
minNode = q;
}
q = q->next;
}
if (minNode != p) {
ListNode* next = minNode->next;
minNode->next = p->next;
p->next = minNode;
swap(minNode->val, p->val);
}
p = p->next;
}
ListNode* newHead = dummy->next;
delete dummy;
return newHead;
}
// 按照指定顺序打印链表
void printList(ListNode* head) {
while (head) {
cout << head->val << " ";
head = head->next;
}
cout << endl;
}
// 随机生成链表
ListNode* generateList(int n) {
srand(time(NULL));
ListNode* dummy = new ListNode(-1);
ListNode* p = dummy;
for (int i = 0; i < n; i++) {
p->next = new ListNode(rand() % 100);
p = p->next;
}
ListNode* head = dummy->next;
delete dummy;
return head;
}
// 释放链表
void freeList(ListNode* head) {
while (head) {
ListNode* next = head->next;
delete head;
head = next;
}
}
// 测试排序算法的正确性
void testSort() {
ListNode* head = new ListNode(5);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(1);
head->next->next->next->next = new ListNode(4);
cout << "Original List: ";
printList(head);
head = insertionSortList(head);
cout << "Insertion Sort: ";
printList(head);
head = bubbleSortList(head);
cout << "Bubble Sort: ";
printList(head);
head = quickSortList(head);
cout << "Quick Sort: ";
printList(head);
head = selectionSortList(head);
cout << "Selection Sort: ";
printList(head);
freeList(head);
}
// 测试排序算法的性能
void testPerformance() {
int sizes[] = { 10, 100, 1000 };
for (int i = 0; i < 3; i++) {
int size = sizes[i];
cout << "Test for " << size << " elements:" << endl;
ListNode* ascList = generateList(size);
for (int j = 1; j <= size; j++) {
ascList->val = j;
ascList = ascList->next;
}
ListNode* descList = generateList(size);
for (int j = size; j >= 1; j--) {
descList->val = j;
descList = descList->next;
}
ListNode* randList = generateList(size);
clock_t start, end;
double duration;
// 插入排序
start = clock();
insertionSortList(ascList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Insertion Sort (Asc): " << duration << "s" << endl;
start = clock();
insertionSortList(descList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Insertion Sort (Desc): " << duration << "s" << endl;
start = clock();
insertionSortList(randList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Insertion Sort (Rand): " << duration << "s" << endl;
// 冒泡排序
start = clock();
bubbleSortList(ascList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Bubble Sort (Asc): " << duration << "s" << endl;
start = clock();
bubbleSortList(descList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Bubble Sort (Desc): " << duration << "s" << endl;
start = clock();
bubbleSortList(randList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Bubble Sort (Rand): " << duration << "s" << endl;
// 快速排序
start = clock();
quickSortList(ascList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Quick Sort (Asc): " << duration << "s" << endl;
start = clock();
quickSortList(descList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Quick Sort (Desc): " << duration << "s" << endl;
start = clock();
quickSortList(randList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Quick Sort (Rand): " << duration << "s" << endl;
// 简单选择排序
start = clock();
selectionSortList(ascList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Selection Sort (Asc): " << duration << "s" << endl;
start = clock();
selectionSortList(descList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Selection Sort (Desc): " << duration << "s" << endl;
start = clock();
selectionSortList(randList);
end = clock();
duration = (double)(end - start) / CLOCKS_PER_SEC;
cout << "Selection Sort (Rand): " << duration << "s" << endl;
freeList(ascList);
freeList(descList);
freeList(randList);
cout << endl;
}
}
int main() {
testSort();
cout << endl;
testPerformance();
return 0;
}
```
在 `testSort()` 函数中,我们对排序算法进行了简单的测试,验证了算法的正确性。在 `testPerformance()` 函数中,我们对排序算法的性能进行了测试,并输出了各个算法在不同输入数据下的执行时间。同时,我们还可以根据计数器的值来比较关键字比较次数和移动次数,从而验证各种算法的时间复杂度。
阅读全文
相关推荐





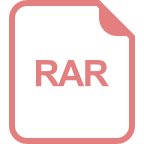
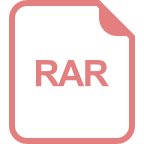
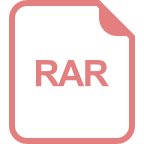
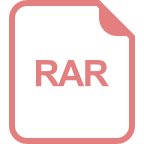








