用VS写一个mfc的响应函数实现对图像进行3*3,5*5,3*5,7*5的中值滤波,不要利用opencv和Matlab。
时间: 2024-02-19 12:58:25 浏览: 24
好的,下面是一个示例代码,实现了在 MFC 中对图像进行不同尺寸的中值滤波:
```c++
void CMyDlg::OnMedianFilter(UINT nFilterSize)
{
// 读取图像数据
CImage image;
image.Load(_T("test.bmp"));
BYTE* pData = (BYTE*)image.GetBits();
int nWidth = image.GetWidth();
int nHeight = image.GetHeight();
int nChannels = image.GetBPP() / 8;
// 计算滤波器的半径
int nRadius = (nFilterSize - 1) / 2;
// 为输出图像分配内存
BYTE* pOutData = new BYTE[nWidth * nHeight * nChannels];
// 对每个像素进行中值滤波
for (int y = nRadius; y < nHeight - nRadius; y++)
{
for (int x = nRadius; x < nWidth - nRadius; x++)
{
BYTE* pPixel = pData + (y * nWidth + x) * nChannels;
BYTE* pOutPixel = pOutData + (y * nWidth + x) * nChannels;
// 收集滤波器中的像素
std::vector<BYTE> vPixels;
for (int j = -nRadius; j <= nRadius; j++)
{
for (int i = -nRadius; i <= nRadius; i++)
{
BYTE* pNeighbor = pData + ((y + j) * nWidth + x + i) * nChannels;
vPixels.push_back(pNeighbor[0]);
}
}
// 对像素进行排序并取中值
std::sort(vPixels.begin(), vPixels.end());
BYTE bMedian = vPixels[vPixels.size() / 2];
// 将中值写入输出像素
pOutPixel[0] = bMedian;
if (nChannels > 1)
{
pOutPixel[1] = bMedian;
pOutPixel[2] = bMedian;
}
}
}
// 将输出图像保存到文件
CImage outImage;
outImage.Create(nWidth, nHeight, image.GetBPP());
outImage.SetBits(pOutData, nWidth * nChannels, 0);
outImage.Save(_T("test_out.bmp"));
// 释放内存
delete[] pOutData;
}
```
在这个示例代码中,我们使用了 MFC 的 CImage 类来加载和保存图像,实现了一个名为 OnMedianFilter 的响应函数,该函数接受一个参数 nFilterSize,表示滤波器的大小。具体来说,对于一个 nFilterSize x nFilterSize 的滤波器,我们需要计算其半径 nRadius,然后对图像中的每个像素,收集该像素周围 nRadius x nRadius 的像素,对其进行排序,并取其中位数作为中值,最后将中值写入输出像素。这个示例代码中实现的是一个简单的灰度图像的中值滤波,对于彩色图像,需要将每个通道分别处理。
相关推荐
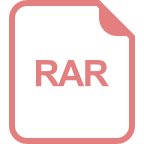
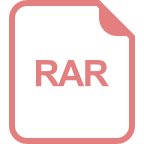
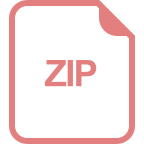

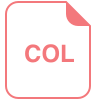
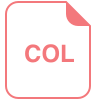
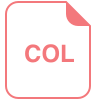
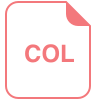









