def func(n): result=[] for i in range(len(n)): if i%2==0: result.append(n[i]) return result l1=[12,2,42,17,27,99,35] print(func(l1))注解每一行,了解每一行代码的含义还有运算
时间: 2024-03-16 07:41:08 浏览: 131
```
1. def func(n): # 定义一个名为 func 的函数,它有一个参数 n
2. result=[] # 初始化一个空列表,用来存储筛选出来的元素
3. for i in range(len(n)): # 遍历 n 中的每一个元素,i 表示当前遍历到的元素的下标
4. if i%2==0: # 如果 i 是偶数
5. result.append(n[i]) # 将 n 中下标为 i 的元素加入到 result 列表中
6. return result # 返回筛选出来的列表
7. l1=[12,2,42,17,27,99,35] # 定义一个列表 l1,用来作为函数 func 的参数
8. print(func(l1)) # 调用函数 func,传入参数 l1,并打印函数的返回结果
```
此代码实现了一个函数,该函数接收一个列表作为参数,筛选出该列表中下标为偶数的元素,并返回筛选出来的列表。在这个例子中,输入的列表为 `[12,2,42,17,27,99,35]`,输出的列表为 `[12, 42, 27, 35]`。
相关问题
import seaborn as sns import matplotlib.pyplot as plt import pandas as pd import numpy as np def factorial(x): result=1 for i in range(1,x+1): result*=i return result sns.set_theme(style="ticks") x=np.array(range(50)) y=np.array([1]*len(x)) y2=np.log2(x) y3=x y4=x*np.log2(x) y5=x**2 y6=x**3 y7=np.power(2,x) y8=np.array(map(factorial,x)) sns.lineplot(x=x,y=y,label='f(n)=1')#折线图 sns.lineplot(x=x,y=y2,label='f(n)=logn') sns.lineplot(x=x,y=y3,label='f(n)=n') sns.lineplot(x=x,y=y4,label='f(n)=nlogn') sns.lineplot(x=x,y=y5,label='f(n)=n^2') sns.lineplot(x=x,y=y6,label='f(n)=n^3') sns.lineplot(x=x,y=y7,label='f(n)=2^n') sns.lineplot(x=x,y=y8,label='f(n)=n!') plt.ylim((0,1500)) plt.savefig("func_plot.pdf", format="pdf", bbox_inches="tight") plt.show() 这段代码哪里出错了?
这段代码可能存在以下问题:
1. 导入语句存在问题。
应该将所有的导入语句放在同一行,例如:
```
import seaborn as sns, matplotlib.pyplot as plt, pandas as pd, numpy as np
```
2. 函数定义存在问题。
在Python 3.x中,`map()`函数返回的是一个迭代器,需要使用`list()`函数将其转换为列表。因此,函数定义应该修改为:
```
def factorial(x):
result = 1
for i in range(1, x+1):
result *= i
return result
y8 = np.array(list(map(factorial, x)))
```
3. 函数调用存在问题。
在使用`np.power()`函数计算2的x次方时,应该将第一个参数设置为2,而不是x。因此,函数调用应该修改为:
```
y7 = np.power(2, x)
```
4. 图形显示存在问题。
在使用`plt.ylim()`函数设置y轴范围时,取值范围过小,可能导致一些线条被遮挡。应该将y轴范围扩大,例如:
```
plt.ylim((0, 20000))
```
综上所述,完整的代码应该修改为:
```
import seaborn as sns, matplotlib.pyplot as plt, pandas as pd, numpy as np
def factorial(x):
result = 1
for i in range(1, x+1):
result *= i
return result
sns.set_theme(style="ticks")
x = np.array(range(50))
y = np.array([1]*len(x))
y2 = np.log2(x)
y3 = x
y4 = x*np.log2(x)
y5 = x**2
y6 = x**3
y7 = np.power(2, x)
y8 = np.array(list(map(factorial, x)))
sns.lineplot(x=x, y=y, label='f(n)=1')
sns.lineplot(x=x, y=y2, label='f(n)=logn')
sns.lineplot(x=x, y=y3, label='f(n)=n')
sns.lineplot(x=x, y=y4, label='f(n)=nlogn')
sns.lineplot(x=x, y=y5, label='f(n)=n^2')
sns.lineplot(x=x, y=y6, label='f(n)=n^3')
sns.lineplot(x=x, y=y7, label='f(n)=2^n')
sns.lineplot(x=x, y=y8, label='f(n)=n!')
plt.ylim((0, 20000))
plt.savefig("func_plot.pdf", format="pdf", bbox_inches="tight")
plt.show()
```
请用c语言重写这段代码from machine import Pin,Timer,SPI import time hspi = SPI(1, 2500000, sck=Pin(14), mosi=Pin(13), miso=Pin(12), polarity=0) def byte2bin(b): bstr = bin(b)[2:] return '0'(8-len(bstr)) + bstr def rgb2byte(r,g,b): str = byte2bin(g) + byte2bin(r) + byte2bin(b) spistr = ''.join([(lambda s: '011' if s'0' else '001')(x) for x in str]) rgbdim = [int(spistr[i8:i8+8], 2) for i in range(9)] return bytes(rgbdim) rgbbyte = rgb2byte(0xff,0x80,0x3f) print(rgbbyte) rstbyte = bytes([0xff]*16) outbyte = rstbyte+rgbbyte while True: hspi.write(outbyte) time.sleep_ms(10)
#include <stdio.h>
#include <stdlib.h>
#include "pico/stdlib.h"
#include "hardware/spi.h"
#include "hardware/gpio.h"
#define SCK_PIN 14
#define MOSI_PIN 13
#define MISO_PIN 12
void byte2bin(uint8_t b, char* result) {
char* bstr = itoa(b, result, 2);
int len = strlen(bstr);
for (int i = 0; i < 8 - len; i++) {
result[i] = '0';
}
result[8 - len] = '\0';
}
void rgb2byte(uint8_t r, uint8_t g, uint8_t b, uint8_t* rgbdim) {
char str[25];
char spistr[25];
char result[9];
byte2bin(g, result);
strcpy(str, result);
byte2bin(r, result);
strcat(str, result);
byte2bin(b, result);
strcat(str, result);
for (int i = 0; i < 24; i++) {
spistr[i] = (str[i] == '0') ? '0' : '1';
}
spistr[24] = '\0';
for (int i = 0; i < 9; i++) {
rgbdim[i] = strtol(spistr + i * 8, NULL, 2);
}
}
int main() {
stdio_init_all();
spi_init(spi1, 2500000);
gpio_set_function(SCK_PIN, GPIO_FUNC_SPI);
gpio_set_function(MOSI_PIN, GPIO_FUNC_SPI);
gpio_set_function(MISO_PIN, GPIO_FUNC_SPI);
uint8_t rgbbyte[9];
rgb2byte(0xff, 0x80, 0x3f, rgbbyte);
uint8_t rstbyte[16];
memset(rstbyte, 0xff, sizeof(rstbyte));
uint8_t outbyte[25];
memcpy(outbyte, rstbyte, sizeof(rstbyte));
memcpy(outbyte + sizeof(rstbyte), rgbbyte, sizeof(rgbbyte));
while (true) {
spi_write_blocking(spi1, outbyte, sizeof(outbyte));
sleep_ms(10);
}
return 0;
}
阅读全文
相关推荐
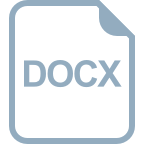
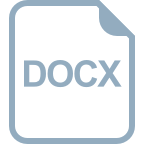
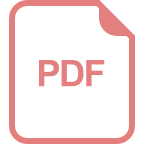
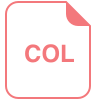
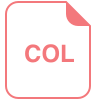
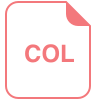
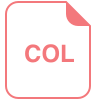
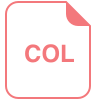
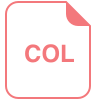
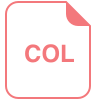
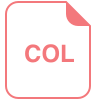
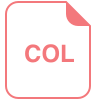
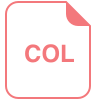
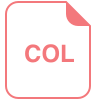



