c++学生类Student中包含当前学生对象的姓名、学号和三门课程的成绩,学生类Student中包含当前学生对象的姓名、学号和三门课程的成绩,在主函数中要求用户输入学生人数num,并根据输入的学生人数num创建动态对象数组。
时间: 2023-12-03 17:47:21 浏览: 73
好的,你可以参考下面的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name; // 学生姓名
string id; // 学生学号
int score1; // 课程1成绩
int score2; // 课程2成绩
int score3; // 课程3成绩
public:
// 构造函数
Student(string n, string i, int s1, int s2, int s3) {
name = n;
id = i;
score1 = s1;
score2 = s2;
score3 = s3;
}
// 输出学生信息
void printInfo() {
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "成绩1:" << score1 << endl;
cout << "成绩2:" << score2 << endl;
cout << "成绩3:" << score3 << endl;
}
};
int main() {
int num; // 学生人数
cout << "请输入学生人数:";
cin >> num;
// 动态创建对象数组
Student *stuArr = new Student[num];
// 循环输入学生信息
for (int i = 0; i < num; i++) {
string name, id;
int score1, score2, score3;
cout << "请输入第" << i+1 << "个学生的姓名:";
cin >> name;
cout << "请输入第" << i+1 << "个学生的学号:";
cin >> id;
cout << "请输入第" << i+1 << "个学生的成绩1:";
cin >> score1;
cout << "请输入第" << i+1 << "个学生的成绩2:";
cin >> score2;
cout << "请输入第" << i+1 << "个学生的成绩3:";
cin >> score3;
stuArr[i] = Student(name, id, score1, score2, score3);
}
// 循环输出学生信息
for (int i = 0; i < num; i++) {
cout << "第" << i+1 << "个学生的信息:" << endl;
stuArr[i].printInfo();
}
// 释放对象数组内存
delete[] stuArr;
return 0;
}
```
代码解释:
1. 定义 `Student` 类,包括姓名、学号和三门课程成绩,以及构造函数和输出学生信息的方法。
2. 在 `main` 函数中,先输入学生人数 `num`。
3. 动态创建 `Student` 对象数组 `stuArr`,长度为 `num`。
4. 循环输入每个学生的信息,并将其保存在相应的 `Student` 对象中。
5. 循环输出每个学生的信息。
6. 释放动态创建的对象数组内存。
阅读全文
相关推荐
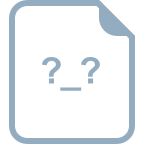
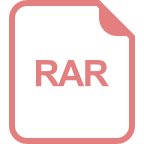
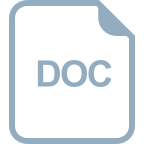















