使用pymysql连接数据库
时间: 2023-10-24 20:16:43 浏览: 57
可以使用pymysql模块来连接MySQL数据库。以下是一个示例代码,演示如何连接MySQL数据库并执行查询:
```python
import pymysql
# 连接到数据库
conn = pymysql.connect(
host='localhost',
user='root',
password='password',
db='test_db',
charset='utf8mb4'
)
# 使用cursor()方法创建一个游标对象
cursor = conn.cursor()
# 执行查询
sql = "SELECT * FROM users"
cursor.execute(sql)
# 获取查询结果
result = cursor.fetchall()
for row in result:
print(row)
# 关闭游标和连接
cursor.close()
conn.close()
```
在此示例中,我们首先使用`pymysql.connect()`方法连接到数据库。然后,我们使用`cursor()`方法创建一个游标对象,该对象用于执行查询。执行查询后,我们使用`fetchall()`方法获取所有结果,并使用循环打印每一行。最后,我们关闭游标和连接。
相关问题
使用pymysql连接数据库blog
好的,以下是连接 MySQL 数据库并操作 blog 数据库的示例代码:
```python
import pymysql
# 数据库连接
connection = pymysql.connect(
host='localhost',
user='root',
password='password',
db='blog',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor
)
try:
with connection.cursor() as cursor:
# 读取数据的 SQL 语句
sql = "SELECT * FROM `posts` WHERE `status`=%s"
cursor.execute(sql, ('published',))
result = cursor.fetchall()
print(result)
finally:
# 关闭数据库连接
connection.close()
```
其中,`host`、`user`、`password`、`db` 分别代表数据库的主机地址、用户名、密码和要操作的数据库名称。`charset` 指定字符集为 `utf8mb4`,可以支持存储 emoji 表情等特殊字符。`cursorclass` 指定游标类型为 `DictCursor`,可以以字典形式返回查询结果。
以上代码使用 `pymysql.connect()` 函数连接到 MySQL 数据库,并使用 `with` 语句创建一个游标对象 `cursor`,然后执行 `SELECT` 语句查询 `posts` 表中状态为 `published` 的所有记录,并将结果以字典形式存储在 `result` 变量中。最后,使用 `finally` 语句关闭数据库连接。
你可以根据自己的需求,修改 SQL 语句和参数,来实现不同的数据库操作。
pymysql连接数据库
在Flask中使用PyMySQL连接数据库的步骤如下:
1. 安装PyMySQL库:可以使用pip install pymysql命令进行安装。
2. 在Flask应用中导入PyMySQL库:可以使用import pymysql语句进行导入。
3. 创建数据库连接:使用pymysql.connect()函数创建数据库连接对象,需要传入数据库的主机名、用户名、密码、数据库名等参数。
4. 创建游标对象:使用连接对象的cursor()方法创建游标对象,用于执行SQL语句。
5. 执行SQL语句:使用游标对象的execute()方法执行SQL语句。
6. 提交事务:使用连接对象的commit()方法提交事务。
7. 关闭游标和连接:使用游标对象的close()方法和连接对象的close()方法关闭游标和连接。
示例代码如下:
```python
import pymysql
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
# 创建数据库连接
conn = pymysql.connect(host='localhost', user='root', password='123456', database='test', charset='utf8')
# 创建游标对象
cursor = conn.cursor()
# 执行SQL语句
cursor.execute('select * from user')
# 获取查询结果
result = cursor.fetchall()
# 提交事务
conn.commit()
# 关闭游标和连接
cursor.close()
conn.close()
return str(result)
if __name__ == '__main__':
app.run()
```
在上面的示例中,我们创建了一个Flask应用,并在路由函数中使用PyMySQL连接数据库,执行了一个查询语句,并将查询结果返回给客户端。注意,在实际开发中,我们应该将数据库连接的创建和关闭放在try...finally语句中,以确保连接和游标对象能够被正确关闭。
相关推荐
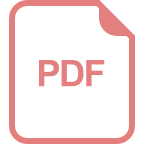
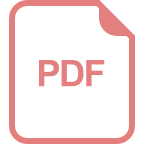











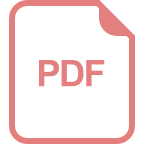