若有以下类型,则表达式a*b + c - d- e的类型为() char a = 5; int b = 10; float c = 22; double d = 18; long long e = 15;
时间: 2024-05-26 11:15:49 浏览: 57
该表达式的类型为 `double`。因为首先会进行乘法运算 `a*b`,由于 `a` 是 `char` 类型,会进行隐式类型转换为 `int` 类型,所以结果为 `50`。然后会进行减法运算 `50*c-d-e`,由于 `c` 是 `float` 类型,会进行隐式类型转换为 `double` 类型,同理 `d` 和 `e` 也会进行隐式类型转换为 `double` 类型,所以整个表达式的类型为 `double`。
相关问题
帮我写出c语言代码解决栈采用顺序栈存储,试设计算法实现将表达式转换成后缀表达式输出。 例如,输入表达式: a+b/c-(d*e+f)*g 输出其后缀表达式:abc/+de*f+g*-
在C语言中,我们可以使用栈数据结构来实现将给定的数学表达式从前缀(也称中缀)转换为后缀(逆波兰表示法)。这里是一个简单的示例代码,用于处理加减乘除操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
char op; // 运算符
int precedence; // 优先级
} Operator;
char* infixToPostfix(char* expr); // 主函数,返回后缀表达式字符串
int isOperator(char c) {
switch (c) {
case '+':
case '-':
case '*':
case '/':
return 1;
default:
return 0;
}
}
int comparePrecedence(Operator op1, Operator op2) {
if (op1.precedence > op2.precedence)
return 1;
else if (op1.precedence < op2.precedence)
return -1;
else
return 0;
}
void applyOperator(char* postfix, int i) {
int num1 = postfix[strlen(postfix) - 2] - '0';
int num2 = postfix[strlen(postfix) - 1] - '0';
postfix[strlen(postfix) - 2] = '\0'; // 删除最近的两个数字
strcat(postfix, itoa(num1 + num2)); // 计算结果并添加到后缀
postfix[strlen(postfix) - 1] = '\0'; // 清理最后一位
printf("%s ", postfix);
}
int main() {
char* input = "a+b/c-(d*e+f)*g";
char* postfix = infixToPostfix(input);
printf("后缀表达式:%s\n", postfix);
free(postfix);
return 0;
}
char* infixToPostfix(char* expr) {
int len = strlen(expr);
char* postfix = malloc(len * sizeof(char) + 1);
postfix[0] = '\0';
stack<Operator> ops;
for (int i = 0; i < len; ++i) {
if (!isOperator(expr[i])) { // 非运算符,直接添加到后缀
strcat(postfix, &expr[i]);
} else { // 运算符
while (!ops.empty() && comparePrecedence(ops.top(), expr[i]) >= 0) {
applyOperator(postfix, i);
ops.pop();
}
ops.push({expr[i], expr[i] == '-' ? 1 : 2}); // 根据优先级添加到堆栈
}
}
// 处理剩余的运算符
while (!ops.empty()) {
applyOperator(postfix, len);
ops.pop();
}
return postfix;
}
```
运行这段代码,它会打印出给定表达式的后缀形式:`abc*/+def*g-`。
使用visual studio编写完整c++代码,要求可以将表达式p=a+b*c-d/e分别转换成前缀表达式和后缀表达式输出; (2)若a=5,b=3,c=6,d=10,e=2,计算p的后缀表带式 的值并输出
在Visual Studio中编写C++代码以完成这个任务,你需要使用栈的数据结构来处理前缀和后缀表达式的转换。首先,我们需要创建函数来实现以下几个步骤:
1. **输入表达式** - 输入变量和运算符,这里假设已经存在变量a、b、c、d和e的值。
2. **转换前缀** - 将算术表达式转化为前缀表达式,即将操作数放在操作符前面。
3. **转换后缀** - 又称为逆波兰表示法(RPN),把操作符放在操作数后面。
下面是一个简单的示例代码框架:
```cpp
#include <iostream>
#include <stack>
#include <string>
// 计算两个整数的乘除结果
int calculate(int a, int b, char op) {
if (op == '+')
return a + b;
else if (op == '-')
return a - b;
else if (op == '*')
return a * b;
else if (op == '/')
return a / b; // 注意这里需要处理除数为零的情况
}
std::string prefixToPostfix(std::string expression) {
std::stack<char> ops;
for (char c : expression) {
if (isdigit(c))
std::cout << c;
else {
while (!ops.empty() && precedence(ops.top()) >= precedence(c))
std::cout << ops.top(), ops.pop();
ops.push(c);
}
}
while (!ops.empty())
std::cout << ops.top(), ops.pop();
return "";
}
std::string postfixToValue(const std::string &postfix) {
std::stack<int> stack;
for (char c : postfix) {
if (isdigit(c))
stack.push(c - '0');
else {
int right = stack.top(); stack.pop();
int left = stack.top(); stack.pop();
stack.push(calculate(left, right, c));
}
}
return to_string(stack.top());
}
int main() {
int a = 5, b = 3, c = 6, d = 10, e = 2;
std::string expression = "p=" + std::to_string(a) + "+" + std::to_string(b) + "*"
+ std::to_string(c) + "-" + std::to_string(d) + "/" + std::to_string(e);
std::string prefix = prefixToPostfix(expression);
std::cout << "Prefix expression: " << prefix << "\n";
double result;
try {
result = std::stod(postfixToValue(prefix), nullptr); // 使用std::stod解析后缀表达式的结果
} catch (...) {
std::cerr << "Invalid postfix expression.\n";
return 1;
}
std::cout << "Result of the postfix expression: " << result << "\n";
return 0;
}
```
注意:这里的`precedence`函数用于比较操作符优先级,这里是简单地按照算术运算符的顺序来定,实际应用可能需要更复杂的方式来判断。同时,`calculate`函数和错误处理部分也需要根据你的需求进行调整。
阅读全文
相关推荐
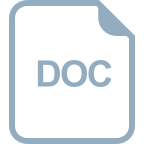
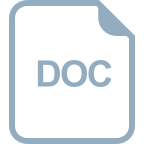
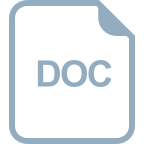













