输入一个中缀表达式,表达式中有+、-、*、/四种运算以及(、),表达式中的其他符号为大写的字母。实现一个算法,得到相应的后缀表达式。C语言实现
时间: 2024-10-20 09:06:49 浏览: 59
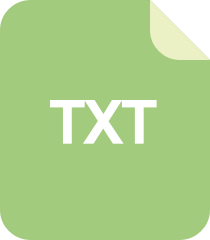
算数表达式的运算(包含+-*/小数,乘方,)

中缀表达式转换成后缀表达式(也称为逆波兰表达式或RPN)的过程通常通过栈数据结构来完成,这种方法被称为Shunting Yard算法。以下是C语言的一个简单实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Token {
enum { NUMBER, PLUS, MINUS, TIMES, DIVIDE, LPAREN, RPAREN } type;
double value; // 对于数字类型的token
} Token;
void process(char *input, char **output) {
Stack *stack = createStack();
char *current = input;
while (*current != '\0') {
if (isdigit(*current)) {
int start = current - input;
while (isdigit(*(current + 1))) {
current++;
}
*current++ = '\0';
Token token = {Token.NUMBER, atof(input + start)};
push(stack, &token);
} else if (*current == '+' || *current == '-' || *current == '*' || *current == '/') {
Token operator = {*(++current), 0};
push(stack, &operator);
} else if (*current == '(') {
push(stack, &{'('});
} else if (*current == ')') {
Token top = pop(stack);
while (!isEmpty(stack) && stack->top.type != '(') {
append(output, &top);
top = pop(stack);
}
if (!isEmpty(stack) && stack->top.type == '(') {
pop(stack);
}
}
}
while (!isEmpty(stack)) {
append(output, pop(stack));
}
}
// 其他辅助函数如创建和销毁栈、处理堆栈操作等
int main() {
char expression[] = "A+B*C-D/(E+F)";
char *postfix = (char*)malloc(sizeof(char) * strlen(expression) * 2); // 略大于原字符串长度用于存储后缀表达式
process(expression, &postfix);
printf("后缀表达式: %s\n", postfix);
free(postfix);
return 0;
}
```
这个程序首先定义了一个`Token`结构体表示输入的不同类型,然后实现了`process`函数来遍历输入的中缀表达式并将其转换为后缀表达式。主函数中提供了一个示例表达式,并打印出转换后的后缀表达式。
阅读全文
相关推荐
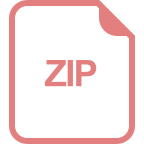















