使用C语言完成下题,逆波兰式也叫后缀表达式(将运算符写在操作数之后) 如:我们平时写a+b,这是中缀表达式,写成后缀表达式就是:ab+ ,使用逆波兰式表达式便于使用栈计算值 输入形式为一行token,使用空格分开,只有 +-*/ 或数字, 使用 @结尾
时间: 2024-12-06 10:26:23 浏览: 29
在C语言中,处理逆波兰式(后缀表达式)通常涉及到两个主要步骤:输入处理和栈操作。这里是一个简单的示例,展示如何使用栈来计算逆波兰式的值:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TOKENS 100
#define MAX_STACK 50
int is_number(char c);
void push(int num, int stack[MAX_STACK]);
int pop(stack_t *s);
double calculate_postfix(char tokens[], char* end);
typedef struct {
int top;
double values[MAX_STACK];
} stack_t;
int is_number(char c) {
return (c >= '0' && c <= '9');
}
void push(int num, int stack[MAX_STACK]) {
if (stack->top < MAX_STACK) {
stack->values[stack->top++] = num;
} else {
printf("Stack overflow!\n");
exit(1);
}
}
int pop(stack_t *s) {
if (s->top > 0) {
return s->values[--s->top];
} else {
printf("Stack underflow!\n");
exit(1);
}
}
double calculate_postfix(char tokens[], char* end) {
stack_t stack;
stack.top = -1;
for (char* token = tokens; token < end; ++token) {
if (is_number(*token)) {
push(strtol(token, NULL, 10), &stack);
} else {
double operand2 = pop(&stack);
double operand1 = pop(&stack);
switch (*token) {
case '+':
stack.values[stack.top] = operand1 + operand2;
break;
case '-':
stack.values[stack.top] = operand1 - operand2;
break;
case '*':
stack.values[stack.top] = operand1 * operand2;
break;
case '/':
stack.values[stack.top] = operand1 / operand2;
break;
}
}
}
// 检查是否只剩下一个元素,即最终结果
if (stack.top != 0) {
printf("Invalid expression!\n");
exit(1);
}
return stack.values[0];
}
int main() {
char input[MAX_TOKENS];
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // Remove newline at the end
char* end = strchr(input, '@');
if (!end) {
printf("Missing terminator '@'\n");
return 1;
}
*end = '\0';
printf("%.2lf\n", calculate_postfix(input, end + 1));
return 0;
}
```
在这个程序中,我们首先检查输入是否有效,然后通过循环遍历tokens,如果遇到数字就压入栈,如果遇到运算符则弹出最近的两个数字进行相应的运算,并将结果压回栈。最后,确保栈为空表示计算成功,返回结果。
阅读全文
相关推荐
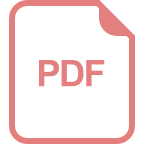
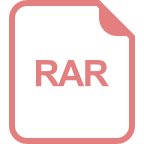
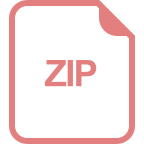





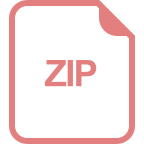
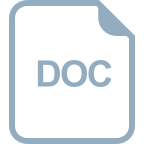
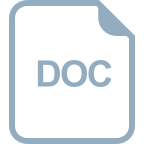
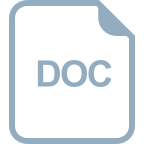
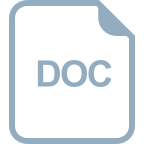





