设表达式由单字母变量、双目运算符和圆括号组成(如:“(a*(b+c)-d)/e)”。 试写一个类C语言,将一个书写正确的表达式转换为逆波兰式
时间: 2024-10-10 07:05:59 浏览: 29
在 C 语言中,我们可以创建一个函数来实现从中缀表达式到后缀(逆波兰)表达式的转换。这里是一个简单的例子,使用栈数据结构来完成这个过程。我们将使用两个指针分别指向当前字符和操作数的位置,并处理遇到的不同情况:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TOKENS 100
typedef struct {
char *token;
int num;
} Token;
Token tokenize(char* expr) {
Token tokens[MAX_TOKENS];
int i = 0;
for (int j = 0; expr[j] != '\0'; ++j) {
if (expr[j] == ' ') continue;
else if (expr[j] >= 'a' && expr[j] <= 'z') {
tokens[i].token = &expr[j];
tokens[i++].num = -1; // 表示这是一个字母操作数
} else if (expr[j] == '+' || expr[j] == '-' || expr[j] == '*' || expr[j] == '/') {
tokens[i].token = &expr[j];
tokens[i++].num = 1; // 表示这是一个运算符
} else if (expr[j] == '(') {
tokens[i].token = &expr[j];
tokens[i++].num = -2; // 表示左括号
} else if (expr[j] == ')') {
tokens[i].token = &expr[j];
tokens[i++].num = 2; // 表示右括号
}
}
return tokens[i]; // 返回最后一个元素作为结束标记
}
char* infix_to_postfix(char* expr) {
Stack stack;
init_stack(&stack);
Token current_token = tokenize(expr);
while (current_token.token != NULL) {
if (current_token.num == -1) { // 字母操作数,直接压入栈
push_stack(stack, current_token.token);
} else if (current_token.num == 1) { // 运算符
while (!is_empty(stack) && precedence(current_token) <= precedence(pop_stack(stack))) {
append_to_result(postfix(stack));
}
push_stack(stack, current_token.token);
} else if (current_token.num == 2) { // 右括号
while (!is_empty(stack) && top_of_stack(stack).num != -2) {
append_to_result(postfix(stack));
pop_stack(stack);
}
pop_stack(stack); // 出去左括号
}
current_token = tokenize(expr);
}
while (!is_empty(stack)) {
append_to_result(postfix(stack));
}
return result_string;
}
// 其他辅助函数省略,例如初始化栈、判断优先级等
void main() {
char expr[] = "(a*(b+c)-d)/e";
char* postfix_expr = infix_to_postfix(expr);
printf("原表达式: %s\n", expr);
printf("后缀表达式: %s\n", postfix_expr);
free(postfix_expr);
}
阅读全文
相关推荐
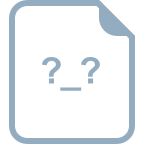
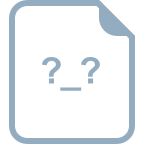
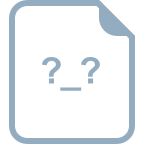


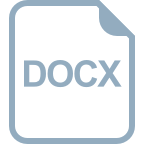
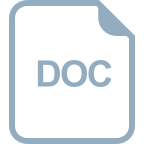
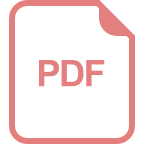
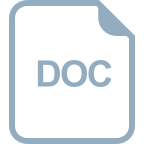
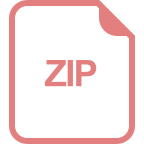
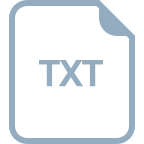
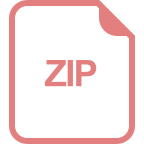
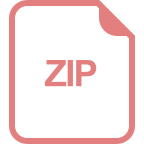
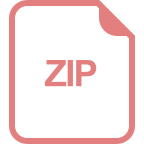
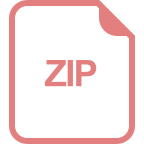