用swift 画一个笑脸 😄
时间: 2023-08-31 13:23:21 浏览: 152
### 回答1:
可以使用 Core Graphics 在 iOS 上画出一个笑脸。下面是一个示例代码:
```
import UIKit
class SmileView: UIView {
override func draw(_ rect: CGRect) {
let facePath = UIBezierPath(arcCenter: CGPoint(x: bounds.midX, y: bounds.midY), radius: bounds.width / 2, startAngle: 0, endAngle: CGFloat.pi * 2, clockwise: true)
facePath.lineWidth = 5.0
UIColor.yellow.setFill()
facePath.fill()
let leftEyePath = UIBezierPath(arcCenter: CGPoint(x: bounds.midX - bounds.width / 4, y: bounds.midY - bounds.width / 4), radius: bounds.width / 10, startAngle: 0, endAngle: CGFloat.pi * 2, clockwise: true)
leftEyePath.lineWidth = 5.0
UIColor.black.setFill()
leftEyePath.fill()
let rightEyePath = UIBezierPath(arcCenter: CGPoint(x: bounds.midX + bounds.width / 4, y: bounds.midY - bounds.width / 4), radius: bounds.width / 10, startAngle: 0, endAngle: CGFloat.pi * 2, clockwise: true)
rightEyePath.lineWidth = 5.0
UIColor.black.setFill()
rightEyePath.fill()
let smilePath = UIBezierPath()
smilePath.move(to: CGPoint(x: bounds.midX - bounds.width / 4, y: bounds.midY + bounds.width / 4))
smilePath.addArc(withCenter: CGPoint(x: bounds.midX, y: bounds.midY + bounds.width / 4), radius: bounds.width / 4, startAngle: CGFloat.pi / 4, endAngle: CGFloat.pi * 3 / 4, clockwise: true)
smilePath.addLine(to: CGPoint(x: bounds.midX + bounds.width / 4, y: bounds.midY + bounds.width / 4))
smilePath.lineWidth = 5.0
smilePath.stroke()
}
}
```
这段代码创建了一个名为 SmileView 的 UIView 子类,在 `draw(_:)` 方法中画出了一个笑脸。使用这个子类创建一个视图,并将其添加到界面上,就可以看到笑脸了。
### 回答2:
在Swift中,可以使用CoreGraphics框架来绘制一个笑脸的笑容。以下是一个简单的实现示例:
import UIKit
// 创建一个空白的UIView
let canvas = UIView(frame: CGRect(x: 0, y: 0, width: 200, height: 200))
// 绘制笑脸
func drawSmileyFace() {
guard let context = UIGraphicsGetCurrentContext() else { return }
// 设置绘制参数
let lineWidth: CGFloat = 3.0
let lineColor = UIColor.black
let fillColor = UIColor.yellow
// 绘制脸部
let faceRect = CGRect(x: 50, y: 50, width: 100, height: 100)
context.setLineWidth(lineWidth)
context.setStrokeColor(lineColor.cgColor)
context.setFillColor(fillColor.cgColor)
context.addEllipse(in: faceRect)
context.drawPath(using: .fillStroke)
// 绘制眼睛
let eyeRadius: CGFloat = 12.0
let eyeOffset: CGFloat = 25.0
context.setFillColor(lineColor.cgColor)
context.fillEllipse(in: CGRect(x: faceRect.minX + eyeOffset, y: faceRect.midY - eyeOffset, width: eyeRadius, height: eyeRadius))
context.fillEllipse(in: CGRect(x: faceRect.maxX - eyeOffset - eyeRadius, y: faceRect.midY - eyeOffset, width: eyeRadius, height: eyeRadius))
// 绘制嘴巴
let mouthOffset: CGFloat = 10.0
let mouthWidth: CGFloat = 50.0
let mouthHeight: CGFloat = 30.0
let mouthRect = CGRect(x: faceRect.midX - mouthWidth / 2, y: faceRect.maxY - mouthOffset - mouthHeight, width: mouthWidth, height: mouthHeight)
context.setLineWidth(lineWidth)
context.setStrokeColor(lineColor.cgColor)
context.addEllipse(in: mouthRect)
context.drawPath(using: .fillStroke)
}
// 使用drawSmileyFace函数来绘制笑脸
canvas.backgroundColor = UIColor.white
canvas.layer.borderWidth = 1.0
canvas.layer.borderColor = UIColor.black.cgColor
canvas.layer.cornerRadius = 10.0
UIGraphicsBeginImageContextWithOptions(CGSize(width: canvas.bounds.width, height: canvas.bounds.height), true, 0)
drawSmileyFace()
let smileyImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
let smileyImageView = UIImageView(frame: CGRect(x: 0, y: 0, width: canvas.bounds.width, height: canvas.bounds.height))
smileyImageView.image = smileyImage
// 在Playground中查看结果
canvas.addSubview(smileyImageView)
阅读全文
相关推荐
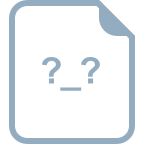
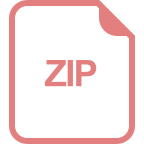














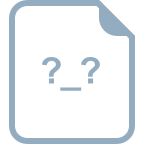