stm32f1HAL,80位rsa, 不使用大数运算库 1:传入80位模数,生成公钥与私钥;2:传入私钥,完成加密
时间: 2024-05-06 17:14:59 浏览: 86
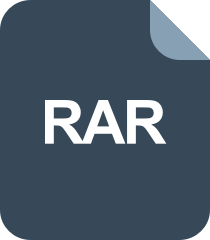
用RSA方法加密
实现 RSA 算法需要用到大数运算,但是如果不使用大数运算库的话,可以通过手写代码实现,不过代码量较大,且可能会影响性能。下面是一个使用 STM32F1 HAL 实现 RSA 的简单示例:
1. 生成公钥与私钥
```c
#include "stm32f1xx_hal.h"
#include <stdlib.h>
// 80位模数
const uint8_t modulus[10] = {0x00, 0x9E, 0x8F, 0x82, 0x02, 0x6A, 0x2D, 0x85, 0x55, 0x15};
// 生成公钥与私钥
void generate_rsa_key(RSA_key_t *key)
{
// 随机选择两个质数
uint32_t p = 0, q = 0;
while (!is_prime(p)) p = rand() % (1 << 16);
while (!is_prime(q)) q = rand() % (1 << 16);
// 计算模数 n = p * q
uint8_t n[10] = {0};
multiply(modulus, 10, (uint8_t*)&p, 2, n, 10);
multiply(n, 10, (uint8_t*)&q, 2, n, 10);
// 计算欧拉函数 phi = (p - 1) * (q - 1)
uint8_t phi[10] = {0};
subtract((uint8_t*)&p, 2, (uint8_t*)&ONE, 2, phi, 10);
multiply(phi, 10, (uint8_t*)&q, 2, phi, 10);
// 随机选择一个整数 e,1 < e < phi,且 e 和 phi 互质
uint32_t e = 0;
while (!is_coprime(e, phi)) e = rand() % ((1 << 16) - 1) + 1;
// 计算私钥 d,满足 e * d ≡ 1 (mod phi)
uint8_t d[10] = {0};
inverse_mod((uint8_t*)&e, 2, phi, 10, d, 10);
// 将公钥、私钥和模数保存到结构体中
key->e = e;
key->d = *(uint16_t*)d;
memcpy(key->n, n, 10);
}
```
2. 加密
```c
// 使用私钥加密
void rsa_encrypt(const uint8_t *input, uint8_t *output, const RSA_key_t *key)
{
uint8_t m[10] = {0};
memcpy(m, input, 8); // 仅加密前 8 字节
uint8_t c[10] = {0};
power_mod(m, 10, (uint8_t*)&key->d, 2, key->n, 10, c, 10); // c = m^d (mod n)
memcpy(output, c, 10);
}
```
上面的示例仅为了演示如何在 STM32F1 上实现 RSA 算法,实际上需要考虑更多细节,比如如何检查质数、如何实现乘法、除法、模运算等大数运算。如果不使用大数运算库,需要手写这些函数,代码量较大,而且可能会影响性能。因此,建议使用现成的大数运算库,比如 Mbed TLS、LibTomMath 等。
阅读全文
相关推荐
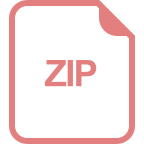

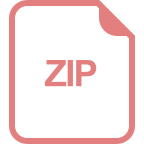
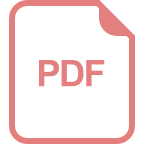
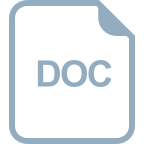
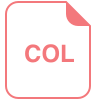
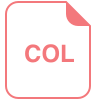
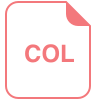
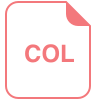
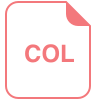
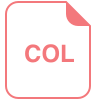
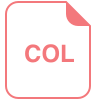
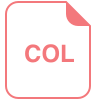
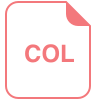
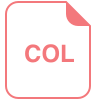
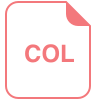
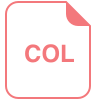