爬取一批相关的英文文献保存到自己的硬盘上,给出python代码
时间: 2024-06-10 21:07:13 浏览: 53
以下是一个简单的Python程序,可用于从网上爬取英文文献并将其保存到本地硬盘上。
```
import requests
import os
def download(url, dir_path):
response = requests.get(url)
filename = os.path.basename(url)
file_path = os.path.join(dir_path, filename)
with open(file_path, 'wb') as f:
f.write(response.content)
if __name__ == '__main__':
url_list = ['https://www.example.com/article1.pdf', 'https://www.example.com/article2.pdf', 'https://www.example.com/article3.pdf']
save_dir = 'papers'
os.makedirs(save_dir, exist_ok=True)
for url in url_list:
download(url, save_dir)
```
本程序使用requests库从网上获取文献,并使用os和os.path模块将他们保存到指定的文件夹中。使用时,只需将待下载文献的URL地址放入列表url_list中,将文件存储目录名保存在save_dir变量中即可。
相关问题
模拟浏览器访问,并爬取网页标题和文本,保存到txt文件,直接写python代码
你好!这是一段使用 Python 爬取网页标题和文本,并保存到 txt 文件的代码:
```python
import requests
from bs4 import BeautifulSoup
# 请求网页
url = 'http://www.example.com'
response = requests.get(url)
# 解析网页
soup = BeautifulSoup(response.text, 'html.parser')
# 提取标题和文本
title = soup.title.string
text = soup.get_text()
# 保存到 txt 文件
with open('result.txt', 'w') as f:
f.write(title + '\n\n')
f.write(text)
print('保存成功!')
```
希望这能帮到你!
python爬取批量图片代码
以下是使用Python爬取批量图片的代码:
```python
import requests
import os
# 创建存储图片的文件夹
if not os.path.exists('images'):
os.makedirs('images')
# 爬取图片
url = 'http://example.com/images/'
for i in range(1, 11):
image_url = url + str(i) + '.jpg'
response = requests.get(image_url)
with open('images/' + str(i) + '.jpg', 'wb') as f:
f.write(response.content)
```
以上代码将从`http://example.com/images/1.jpg`到`http://example.com/images/10.jpg`的所有图片保存在`images`文件夹中。你可以根据自己的需要修改代码来适应不同的爬取需求。
阅读全文
相关推荐
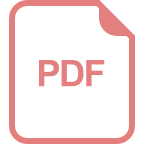
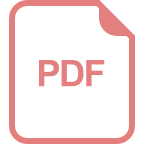
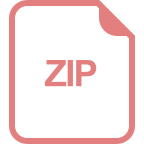
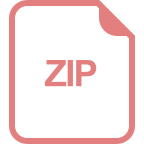
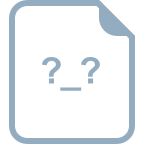
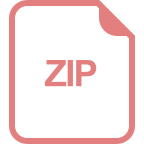



