STM32串口中断同时接收多个字符串
时间: 2023-08-22 12:07:51 浏览: 314
在 STM32 的串口中断中,可以使用循环缓冲区的方式来处理同时接收多个字符串的情况。具体操作步骤如下:
1. 在初始化串口时,开启接收中断,并设置接收中断优先级。
2. 在中断服务函数中,先判断接收中断标志位是否置位,如果是,则将接收到的数据存入循环缓冲区。
3. 在主程序中,通过判断循环缓冲区中是否有完整的字符串,来处理多个字符串的接收。可以使用字符串结束符(例如 '\0')来判断字符串是否接收完整。
4. 处理完整的字符串后,可以将其从循环缓冲区中删除,以便下一个字符串的接收。
以下是一个简单的示例代码:
```c
#define BUF_SIZE 128
char rx_buffer[BUF_SIZE];
int rx_head = 0, rx_tail = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) {
char ch = USART_ReceiveData(USART1);
int next_head = (rx_head + 1) % BUF_SIZE;
if (next_head != rx_tail) {
rx_buffer[rx_head] = ch;
rx_head = next_head;
}
}
}
int main(void)
{
// 初始化串口和循环缓冲区
while (1) {
// 处理完整的字符串
while (rx_head != rx_tail) {
int len = 0;
for (int i = rx_tail; i != rx_head; i = (i + 1) % BUF_SIZE) {
if (rx_buffer[i] == '\0') {
len = i - rx_tail + 1;
break;
}
}
if (len > 0) {
// 处理完整的字符串
// ...
// 从缓冲区中删除字符串
rx_tail += len;
if (rx_tail >= BUF_SIZE) {
rx_tail -= BUF_SIZE;
rx_head -= BUF_SIZE;
}
} else {
// 没有完整的字符串,退出循环
break;
}
}
}
}
```
阅读全文
相关推荐
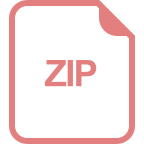

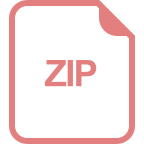
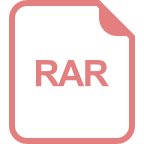
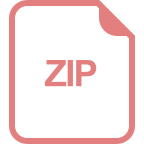
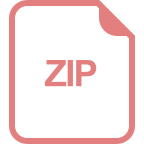
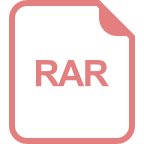
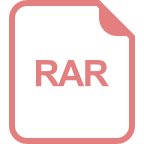
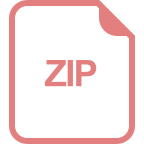
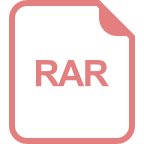
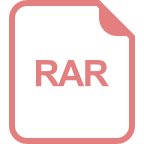
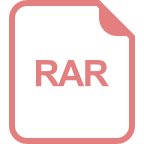
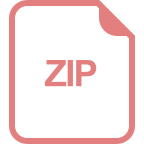
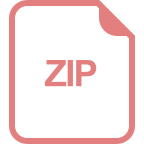
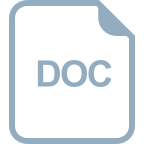
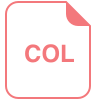
