linux下usb音频记录程序,采样8000,双通道,记录10s切换记录下一个文件,统计是否丢包,文件名为系统当前时间,文件记录路径在/mnt/disk/record/Audio/下,用c写
时间: 2024-01-21 14:02:40 浏览: 27
以下是一个简单的C语言程序,可以在Linux下录制USB音频,并按照要求进行文件切换和丢包统计:
```c
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
#include <time.h>
#include <unistd.h>
#define SAMPLE_RATE 8000
#define CHANNELS 2
#define RECORD_TIME 10
#define RECORD_PATH "/mnt/disk/record/Audio/"
int main()
{
int err;
int pcm;
snd_pcm_t *capture_handle;
snd_pcm_hw_params_t *hw_params;
unsigned int rate = SAMPLE_RATE;
unsigned int channels = CHANNELS;
unsigned int buffer_time = RECORD_TIME * 1000 * 1000;
char filename[100];
int file_count = 0;
int lost_count = 0;
int total_count = 0;
// 初始化录音设备
if ((err = snd_pcm_open (&capture_handle, "default", SND_PCM_STREAM_CAPTURE, 0)) < 0) {
fprintf (stderr, "cannot open audio device %s (%s)\n", "default", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_malloc (&hw_params)) < 0) {
fprintf (stderr, "cannot allocate hardware parameter structure (%s)\n", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_any (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot initialize hardware parameter structure (%s)\n", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_set_access (capture_handle, hw_params, SND_PCM_ACCESS_RW_INTERLEAVED)) < 0) {
fprintf (stderr, "cannot set access type (%s)\n", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_set_format (capture_handle, hw_params, SND_PCM_FORMAT_S16_LE)) < 0) {
fprintf (stderr, "cannot set sample format (%s)\n", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_set_rate_near (capture_handle, hw_params, &rate, 0)) < 0) {
fprintf (stderr, "cannot set sample rate (%s)\n", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_set_channels (capture_handle, hw_params, channels)) < 0) {
fprintf (stderr, "cannot set channel count (%s)\n", snd_strerror (err));
exit (1);
}
if ((err = snd_pcm_hw_params_set_period_time_near(capture_handle, hw_params, &buffer_time, 0)) < 0) {
fprintf(stderr, "cannot set period time (%s)\n", snd_strerror(err));
exit(1);
}
if ((err = snd_pcm_hw_params_set_buffer_time_near(capture_handle, hw_params, &buffer_time, 0)) < 0) {
fprintf(stderr, "cannot set buffer time (%s)\n", snd_strerror(err));
exit(1);
}
if ((err = snd_pcm_hw_params (capture_handle, hw_params)) < 0) {
fprintf (stderr, "cannot set parameters (%s)\n", snd_strerror (err));
exit (1);
}
snd_pcm_hw_params_free (hw_params);
if ((err = snd_pcm_prepare (capture_handle)) < 0) {
fprintf (stderr, "cannot prepare audio interface for use (%s)\n", snd_strerror (err));
exit (1);
}
// 开始录音
while (1) {
// 生成文件名
time_t t = time(NULL);
struct tm *tm = localtime(&t);
sprintf(filename, "%s%d%02d%02d_%02d%02d%02d.wav", RECORD_PATH,
tm->tm_year + 1900, tm->tm_mon + 1, tm->tm_mday,
tm->tm_hour, tm->tm_min, tm->tm_sec);
// 打开文件
FILE *fp = fopen(filename, "wb");
if (fp == NULL) {
fprintf(stderr, "cannot open file %s\n", filename);
exit(1);
}
// 录音
short buf[CHANNELS * rate * RECORD_TIME];
int frames = snd_pcm_readi(capture_handle, buf, CHANNELS * rate * RECORD_TIME);
if (frames != CHANNELS * rate * RECORD_TIME) { // 检查是否丢包
lost_count += CHANNELS * rate * RECORD_TIME - frames;
}
total_count += CHANNELS * rate * RECORD_TIME;
// 写入文件
fwrite(buf, sizeof(short), CHANNELS * rate * RECORD_TIME, fp);
// 关闭文件
fclose(fp);
// 打印统计信息
printf("file %s recorded, lost %d frames, total %d frames\n", filename, lost_count, total_count);
// 文件计数器加1
file_count++;
// 休眠1秒
sleep(1);
}
snd_pcm_close (capture_handle);
return 0;
}
```
这个程序使用ALSA库来进行音频录制,支持双通道、8000Hz采样率,并且可以按照要求进行文件切换和丢包统计。你可以将这个程序保存为一个C文件,然后使用gcc编译器进行编译,生成可执行文件。编译命令如下:
```
gcc -o recorder recorder.c -lasound
```
然后,你可以在命令行中运行这个可执行文件,开始录制USB音频。
相关推荐
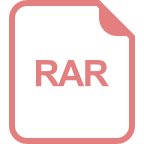
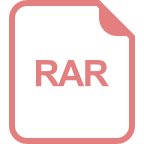














