基于char* 设计一个字符串类MyString,具有构造函数、析构函数、复制构造函数。具体如下: MyString(); MyString(const char* cString); char at(int index) const; int length() const; void clear(); bool empty() const; int compare(const MyString& s) const; int compare(int index, int n, const MyString& s) const; void copy(char s[], int index , int n); char* data() const; int find(char ch) const ; int find(char ch ,int index) const; int find(const MyString& s, int index) const; 另外,还有append、assign、substr、erase函数,详见11.14和11.15要求。
时间: 2024-02-23 14:01:11 浏览: 73
好的,下面是一个可能的实现代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
private:
char* str;
int len;
public:
MyString() : len(0), str(nullptr) {}
MyString(const char* cString) : len(strlen(cString)), str(new char[len + 1]) {
strcpy(str, cString);
}
MyString(const MyString& other) : len(other.len), str(new char[len + 1]) {
strcpy(str, other.str);
}
~MyString() {
if (str != nullptr)
delete[] str;
}
char at(int index) const {
if (index < 0 || index >= len)
throw "Index out of range";
return str[index];
}
int length() const {
return len;
}
void clear() {
if (str != nullptr) {
delete[] str;
str = nullptr;
}
len = 0;
}
bool empty() const {
return len == 0;
}
int compare(const MyString& s) const {
return strcmp(str, s.str);
}
int compare(int index, int n, const MyString& s) const {
if (index < 0 || index >= len || n < 0 || n > len - index)
throw "Invalid index or length";
return strncmp(str + index, s.str, n);
}
void copy(char s[], int index, int n) {
if (index < 0 || index >= len || n < 0 || n > len - index)
throw "Invalid index or length";
strncpy(s, str + index, n);
}
char* data() const {
return str;
}
int find(char ch) const {
return find(ch, 0);
}
int find(char ch, int index) const {
if (index < 0 || index >= len)
throw "Invalid index";
char* p = strchr(str + index, ch);
if (p == nullptr)
return -1;
else
return p - str;
}
int find(const MyString& s, int index) const {
if (index < 0 || index >= len)
throw "Invalid index";
char* p = strstr(str + index, s.str);
if (p == nullptr)
return -1;
else
return p - str;
}
MyString& append(const MyString& other) {
int newLen = len + other.len;
char* newStr = new char[newLen + 1];
strcpy(newStr, str);
strcat(newStr, other.str);
if (str != nullptr)
delete[] str;
str = newStr;
len = newLen;
return *this;
}
MyString& assign(const MyString& other) {
if (this == &other)
return *this;
if (str != nullptr)
delete[] str;
len = other.len;
str = new char[len + 1];
strcpy(str, other.str);
return *this;
}
MyString substr(int index, int n) const {
if (index < 0 || index >= len || n < 0 || n > len - index)
throw "Invalid index or length";
char subStr[n + 1];
strncpy(subStr, str + index, n);
subStr[n] = '\0';
return MyString(subStr);
}
MyString& erase(int index, int n) {
if (index < 0 || index >= len || n < 0 || n > len - index)
throw "Invalid index or length";
char* newStr = new char[len - n + 1];
strncpy(newStr, str, index);
strncpy(newStr + index, str + index + n, len - index - n);
newStr[len - n] = '\0';
if (str != nullptr)
delete[] str;
str = newStr;
len -= n;
return *this;
}
};
int main() {
MyString s1("Hello");
cout << s1.length() << endl; // 5
cout << s1.at(1) << endl; // 'e'
s1.clear();
cout << s1.empty() << endl; // 1
MyString s2("World");
cout << s2.compare(MyString("World")) << endl; // 0
cout << s2.compare(1, 3, MyString("orl")) << endl; // 0
char s[10];
s2.copy(s, 1, 3);
s[3] = '\0';
cout << s << endl; // "orl"
cout << s2.data() << endl; // "World"
cout << s2.find('r') << endl; // 2
cout << s2.find('r', 3) << endl; // 3
cout << s2.find(MyString("rl")) << endl; // 2
s2.append(MyString("!"));
cout << s2.data() << endl; // "World!"
s2.assign(MyString("Hello"));
cout << s2.data() << endl; // "Hello"
MyString s3 = s2.substr(1, 3);
cout << s3.data() << endl; // "ell"
s2.erase(1, 3);
cout << s2.data() << endl; // "Ho"
return 0;
}
```
这个 `MyString` 类中,包含了一个字符指针和一个整数,分别用 `str` 和 `len` 表示。构造函数、复制构造函数和析构函数都有实现。每个成员函数都有一些基本的字符串操作,例如访问指定位置的字符、获取字符串长度、清空字符串、比较字符串、查找指定字符或子串、拷贝指定长度的子串、获取字符串的指针、在末尾追加字符串、替换为指定字符串的拷贝、获取子串等等。在主函数中,使用这些函数进行了一些操作,以便验证它们的正确性。
阅读全文
相关推荐


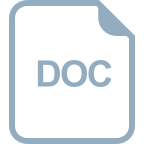















